jQuery Development Tools
As with other languages, jQuery development requires an editor to write a jQuery code and jQuery library.
Editor
You can use any JavaScript editor to write jQuery code including following.
- Notepad
- Visual Studio
- Eclipse
- Aptana Studio
- Ultra edit
An editor which supports IntelliSense for JavaScript and jQuery functions will increase a developer's productivity.
jQuery Library
To download jQuery library, go to jquery.com.
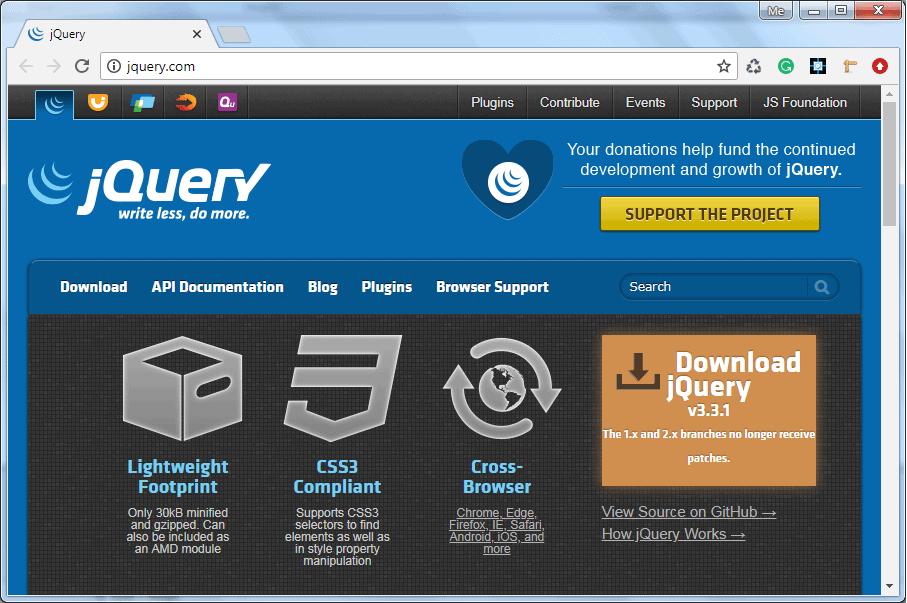
As shown above, click on Download jQuery link or Download menu. This will open download page as shown below.
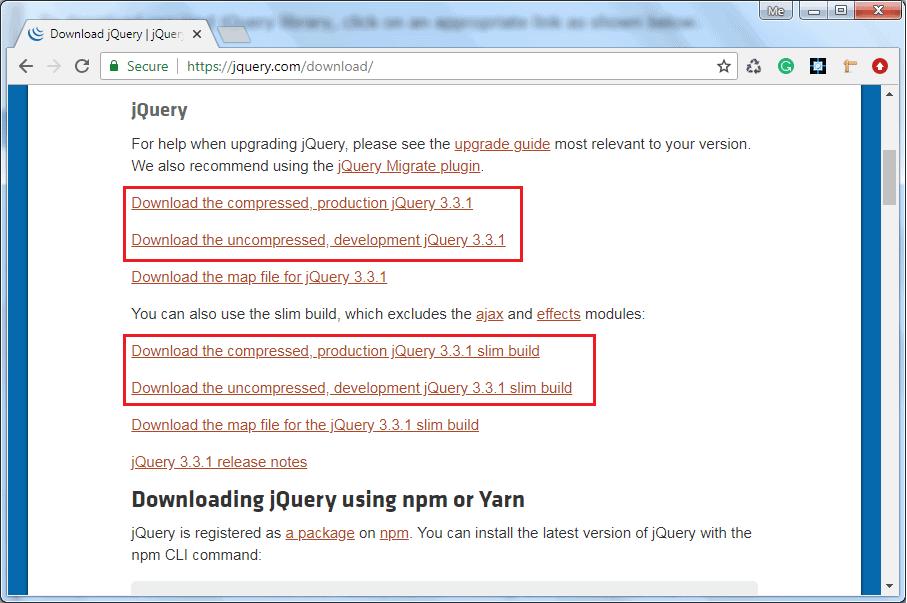
Here, you can download the latest versions of jQuery, at this point it is v3.3. As you can see in the above figure, you can download compressed or uncompressed versions of jQuery library. Compressed version should be used in production environment whereas uncompressed version should be used in development. Compressed version minimizes the library by eliminating extra white space, line feed and shortening the variable and function names. So, compressed version is not readable. Uncompressed library is a readable file which is useful while debugging.
After downloading an appropriate version of jQuery library, you can use it by taking a reference of it in your web page. Remember, jQuery library is eventually a JavaScript file. So you can include it like a normal JavaScript file using script tag as below.
<!DOCTYPE html>
<html>
<head>
<b><script src="~/Scripts/jquery-3.3.1.js"></script> </b>
</head>
<body>
</body>
</html>
Include jQuery Library from CDN
You can also reference jQuery library from public CDN (content delivery network) such as Google, Microsoft, CDNJS, jsDelivr etc.
<!DOCTYPE html>
<html>
<head>
<script src="https://code.jquery.com/jquery-3.3.1.min.js"
integrity="sha256-FgpCb/KJQlLNfOu91ta32o/NMZxltwRo8QtmkMRdAu8="
crossorigin="anonymous">
</script>
</head>
<body>
</body>
</html>
There might be times when these CDN goes down for some reason. Therefore, you need to have a fall back mechanism which downloads jQuery library from your web server if CDN is down.
The following example shows how to include jQuery library reference from CDN with fall back.
<!DOCTYPE html>
<html>
<head>
<script src="https://code.jquery.com/jquery-3.3.1.min.js"
integrity="sha256-FgpCb/KJQlLNfOu91ta32o/NMZxltwRo8QtmkMRdAu8="
crossorigin="anonymous">
</script>
<script>
// Fallback to loading jQuery from a local path if the CDN is unavailable
(window.jQuery || document.write('<script src="~/scripts/jquery-3.3.1.min.js"></script>'));
</script>
</head>
<body>
</body>
</html>
As you can see in the above code example, if jQuery library is successfully loaded then it adds global function jQuery() to window object. If window.jQuery is undefined or null that means jQuery library is not loaded and so you have to download library from local server path.
So, in this way, you can download and take a reference of jQuery library in your web page.
jQuery API Documentation
jQuery provides online API documentation. Click on API Documentation on jquery.com or goto api.jquery.com. You will see a documentation page as shown below.
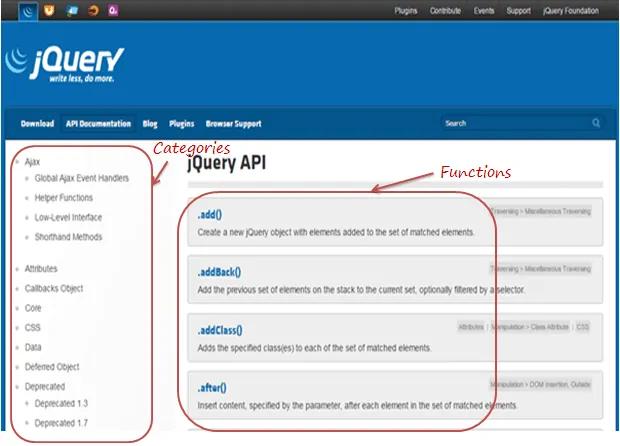
As you can see above, categories of jQuery features are listed on the left side. Right-hand section shows functions related to the currently selected category. Click on a function to get detailed information with example.
After setting up the development environment, let's look at an overview of jQuery syntax and how to get started in the next section.