Create a View in ASP.NET MVC
In this section, you will learn how to create a view and use the model class in it in the ASP.NET MVC application.
A view is used to display data using the model class object. The Views folder contains all the view files in the ASP.NET MVC application.
A controller can have one or more action methods, and each action method can return a different view. In short, a controller can render one or more views. So, for easy maintenance, the MVC framework requires a separate sub-folder for each controller with the same name as a controller, under the Views folder.
For example, all the views rendered from the HomeController
will resides in the Views > Home folder. In the same way, views for StudentController
will resides in Views > Student folder, as shown below.
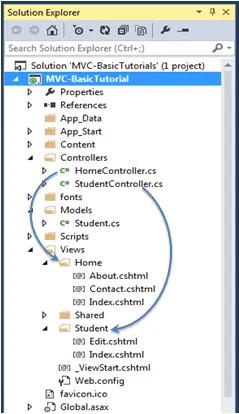
The Shared folder contains views, layout views, and partial views, which will be shared among multiple controllers.
Razor View Engine
Microsoft introduced the razor view engine to compile a view with a mix of HTML tags and server-side code. The special syntax for razor view maximizes the speed of writing code by minimizing the number of characters and keystrokes required when writing a view.
The razor view uses @ character to include the server-side code instead of the traditional <% %>
of ASP. You can use C# or Visual Basic syntax to write server-side code inside the razor view.
ASP.NET MVC supports the following types of razor view files:
File extension | Description |
---|---|
.cshtml | C# Razor view. Supports C# code with html tags. |
.vbhtml | Visual Basic Razor view. Supports Visual Basic code with html tags. |
.aspx | ASP.Net web form |
.ascx | ASP.NET web control |
Learn Razor syntax in the next section.
Creating a View
You can create a view for an action method directly from it by right clicking inside an action method and select Add View...
The following creates a view from the Index()
action method of the StudentContoller
, as shown below.
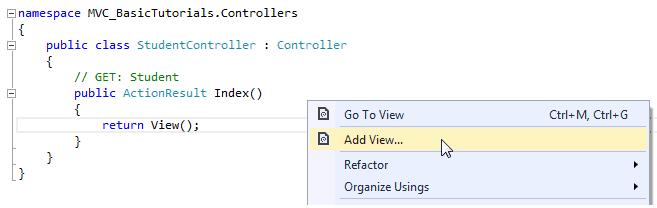
This will open the Add View dialogue box, shown below. It's good practice to keep the view name the same as the action method name so that you don't have to explicitly specify the view name in the action method while returning the view.
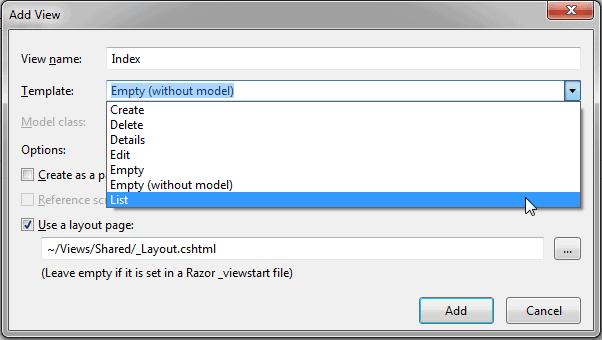
Select the scaffolding template. Template dropdown will show default templates available for Create, Delete, Details, Edit, List, or Empty view. Select "List" template because we want to show the list of students in the view.
Now, select Student
from the model class dropdown. The model class dropdown automatically displays the name of all the classes in the Model
folder. We have already created the Student
model class in the previous section, so it would be included in the dropdown.
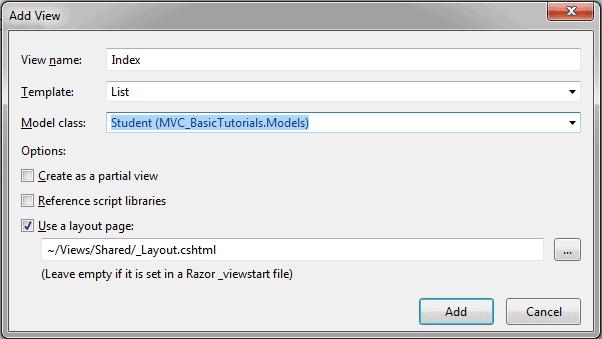
Check "Use a layout page" checkbox and select the default _Layout.cshtml
page for this view and then click Add button.
This will create the Index
view under View -> Student folder, as shown below:
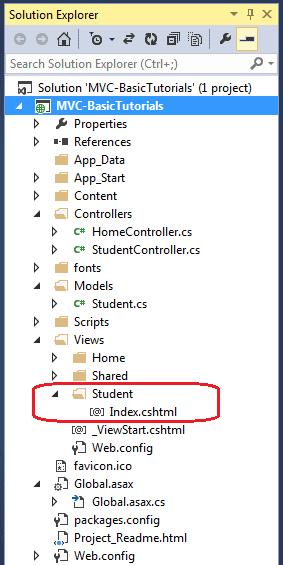
The following code snippet shows an Index.cshtml created above.
@model IEnumerable<MVC_BasicTutorials.Models.Student>
@{
ViewBag.Title = "Index";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Index</h2>
<p>
@Html.ActionLink("Create New", "Create")
</p>
<table className="table">
<tr>
<th>
@Html.DisplayNameFor(model => model.StudentName)
</th>
<th>
@Html.DisplayNameFor(model => model.Age)
</th>
<th></th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.StudentName)
</td>
<td>
@Html.DisplayFor(modelItem => item.Age)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { id=item.StudentId }) |
@Html.ActionLink("Details", "Details", new { id=item.StudentId }) |
@Html.ActionLink("Delete", "Delete", new { id = item.StudentId })
</td>
</tr>
}
</table>
As you can see in the above Index
view, it contains both HTML and razor codes. Inline razor expression starts with @ symbol. @Html is a helper class to generate HTML controls. You will learn razor syntax and HTML helpers in the coming sections.
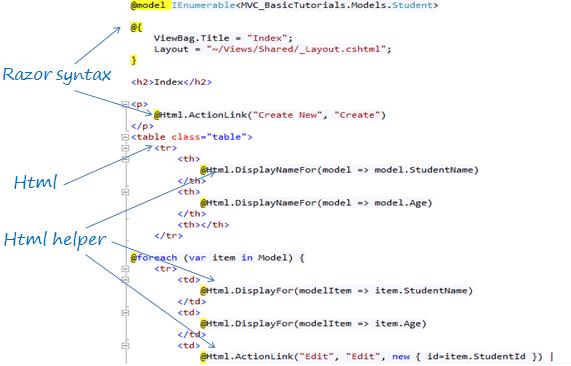
The above Index view would look as below when we run the application.
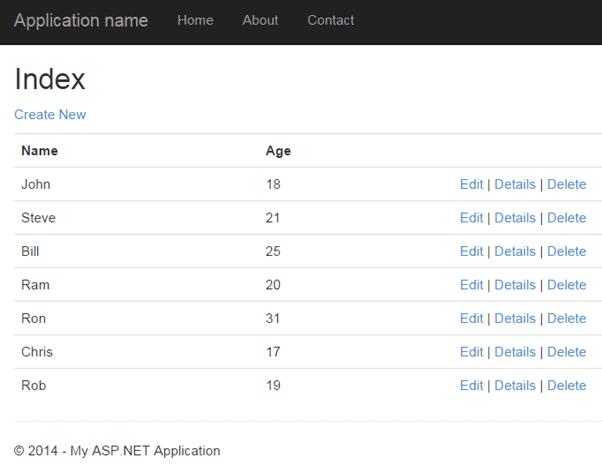
Every view in the ASP.NET MVC is derived from WebViewPage
class included in System.Web.Mvc
namespace.
We need to pass a model object to a view in order to display the data on the view. Learn how to integrate a model, view, and controller in the next chapter.