In C#, the Main() method is an entry point of the Console, Windows, or Web application (.NET Core).
It can have a string[] args
parameter that can be used to retrieve the arguments passed while running the application.
The following example displays the command-line arguments using the args
parameter.
class Program
{
static void Main(string[] args)
{
//program execution starts from here
Console.WriteLine("Total Arguments: {0}", args.Length);
Console.Write("Arguments: ");
foreach (var arg in args)
Console.Write(arg + ", ");
}
}
In the above example, the execution of a console application starts from the Main()
method.
The string[] args
parameter contains values passed from the command-line while executing an application from the command prompt/terminal.
Now, let's run the above program from the command prompt in Windows.
First of all, you have to set your .NET framework path to your environment variable Path
.
This folder is generally C:\Windows\Microsoft.NET\Framework
folder. If you are using .NET Framework 4.x then there will be a folder something like v4.0.30319
based on the version installed on your PC.
Now, open System Properties of your PC by typing "variable" in the search box in Windows 10 and click on "Edit system environment variables". This will open the System Properties window shown below.
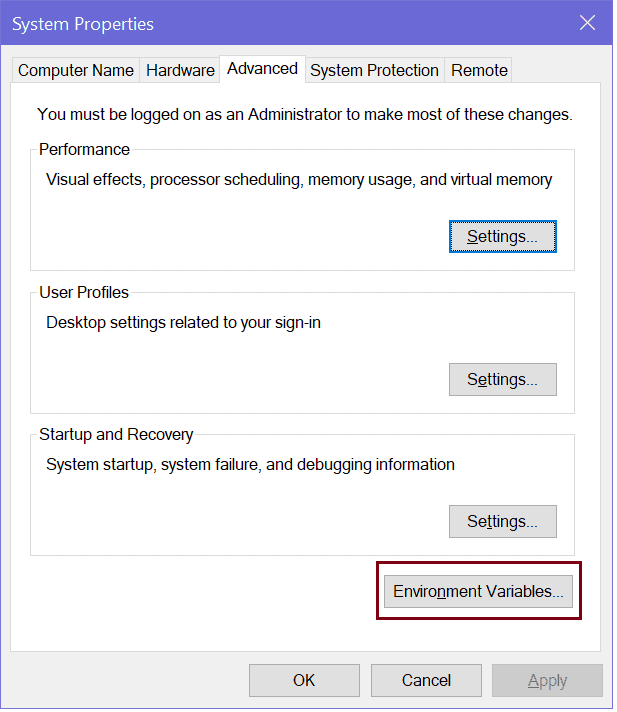
In the above "System Properties" window, click on the Environment Variables..
button to open the Environ Variables window like below.
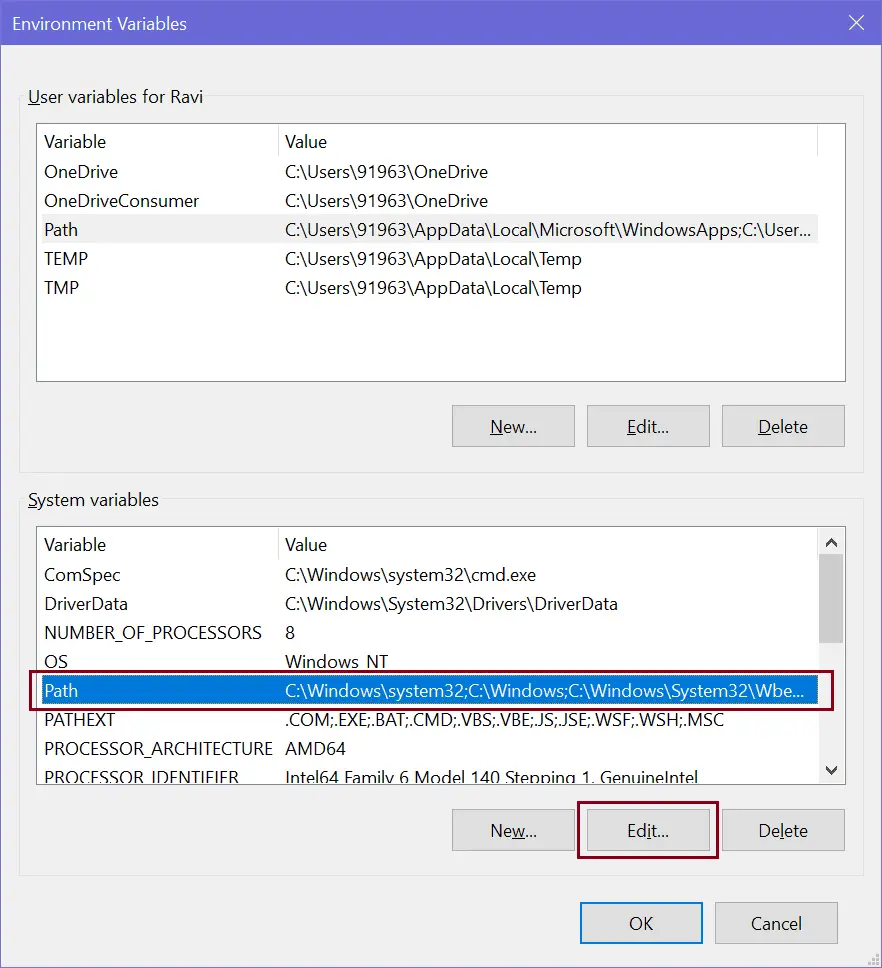
Now, select the Path
variable and click on the Edit button. This will open Edit environment variable window like below.

Click on the New button and add the .NET Framework path and click OK untill you are out.
After setting a Path, open the command prompt and navigate to the folder where you saved your Program.cs
or .cs
file and compile the file using the csc
command, as shown below.
The above command will compile the Program.cs and generate the myprogram.exe
. You can specify any name you like.
Now, to run the application and pass the arguments to the Main()
method, type the program name and specify arguments and press enter, as shown below.
The above command will execute the program and display the following output.
C:\pathtoapp>myprogram.exe "First Arg" 10 20Total Arguments: 3
Arguments: FirstArg, 10, 20,
Thus, you can pass and access the command-line arguments in C# applications.