How to display a custom error page and returning an error code in ASP.NET MVC
You can use the <customErrors>
in web.config to display custom pages when exceptions occurred in MVC application with the response status code 200.
For SEO reason, you may want to display a custom error page and return an appropriate error code in ASP.NET webform or MVC application.
The <customErrors>
section is only used for handling exceptions at the ASP.NET application level. There is an IIS web server between users and the application.
The httpErrors element under <system.webServer>
in web.config is used to configure IIS level errors (IIS 7+). It overrides the configuration of <customErrors>
section.
To display a custom error page with an appropriate error code, use the <httpErrors>
section only, and do not use the <customErrors>
section.
Add the following <httpErrors>
section under <system.webServer>
section, as shown below.
<httpErrors errorMode="Custom" existingResponse="Replace" >
<remove statusCode="500"/>
<error statusCode="500" path="500.html" responseMode="File"/>
<remove statusCode="404"/>
<error statusCode="404" path="404.html" responseMode="File"/>
<remove statusCode="400"/>
<error statusCode="400" path="400.html" responseMode="File"/>
</httpErrors>
The above <httpErrors>
replaces the response coming from ASP.NET with the matching status code and return a custom HTML file as a response. This will preserve the URL and return a custom error page with an appropriate error code.
To make the above config work, create 500.html, 404.html, 400.html at the root of the application. The following is 404.html file.
<!DOCTYPE html>
<html>
<body>
<h1>Page not found.</h1>
</body>
</html>
Now, navigate to a non-existent page of your application, and you will see the following response.
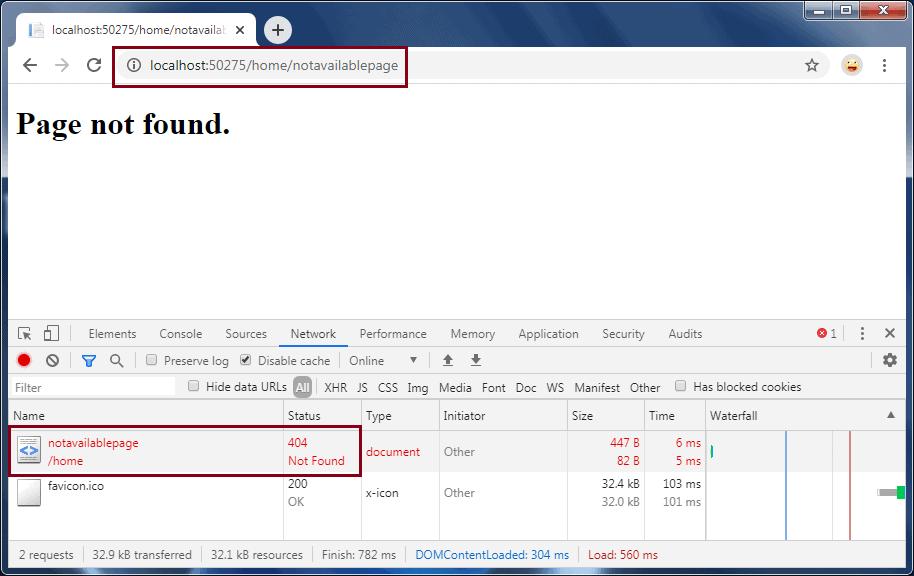
Let's understand the above <httpErrors>
settings.
The errorMode
attribute can have three values: DetailedLocalOnly, Detailed, and Custom. We want to test it on the localhost, so used the Custom
mode. Normally, you should use DetailedLocalOnly
, which will display error info on the localhost but custom error page remotely.
The existingResponse
attribute can have three values: Auto, Replace, and PassThrough. Since we want to return a custom error page with an error code, we will have to replace the error response coming from the ASP.NET application. So, use existingResponse="Replace"
.
<remove statusCode="500" />
removes a specific error message from the collection of error messages your site or application inherits from a higher level in the IIS configuration hierarchy.
<error statusCode="500" path="500.html" responseMode="File" />
configures the 500 error code. The path attribute defines the path to a custom error page to return. The responseMode
attribute specifies whether IIS serves static content, dynamic content, or redirects to a separate URL in response to an error.
The above <httpErrors>
element configures three status code 500, 404, and 400. This will return custom page 500.html, 404.html, and 400.html for the status code 500, 404, and 400, respectively.
Note that you have to create these HTML files in the root folder of the application.
In this way, you can return a custom error page with an appropriate status code to the users in the ASP.NET webform or MVC application.
Learn more about httpErrors here.