Here you will learn how to convert C# object to JSON using Serialization.
JSON (Javascript Object Notation) is used for storing and data transfer. It is also used in API calls to exchange the data from API to different web applications or from browser to server and vice versa.
Serialization is the process of storing the state of an object and being able to recreate it when required. The reverse of it is known as Deserialization.
The .NET 5 framework provides the built-in JsonSerializer
class in the System.Text.Json
namespace to convert C# objects to JSON and vice-versa.
The .NET 4.x framework does not provide any built-in JsonSerializer
class that converts objects to JSON.
You have to install the NuGet package Microsoft.Extensions.Configuration.Json
in your project to include the System.Text.Json.JsonSerializer
to your project which can be used to convert objects to JSON and vice-versa.
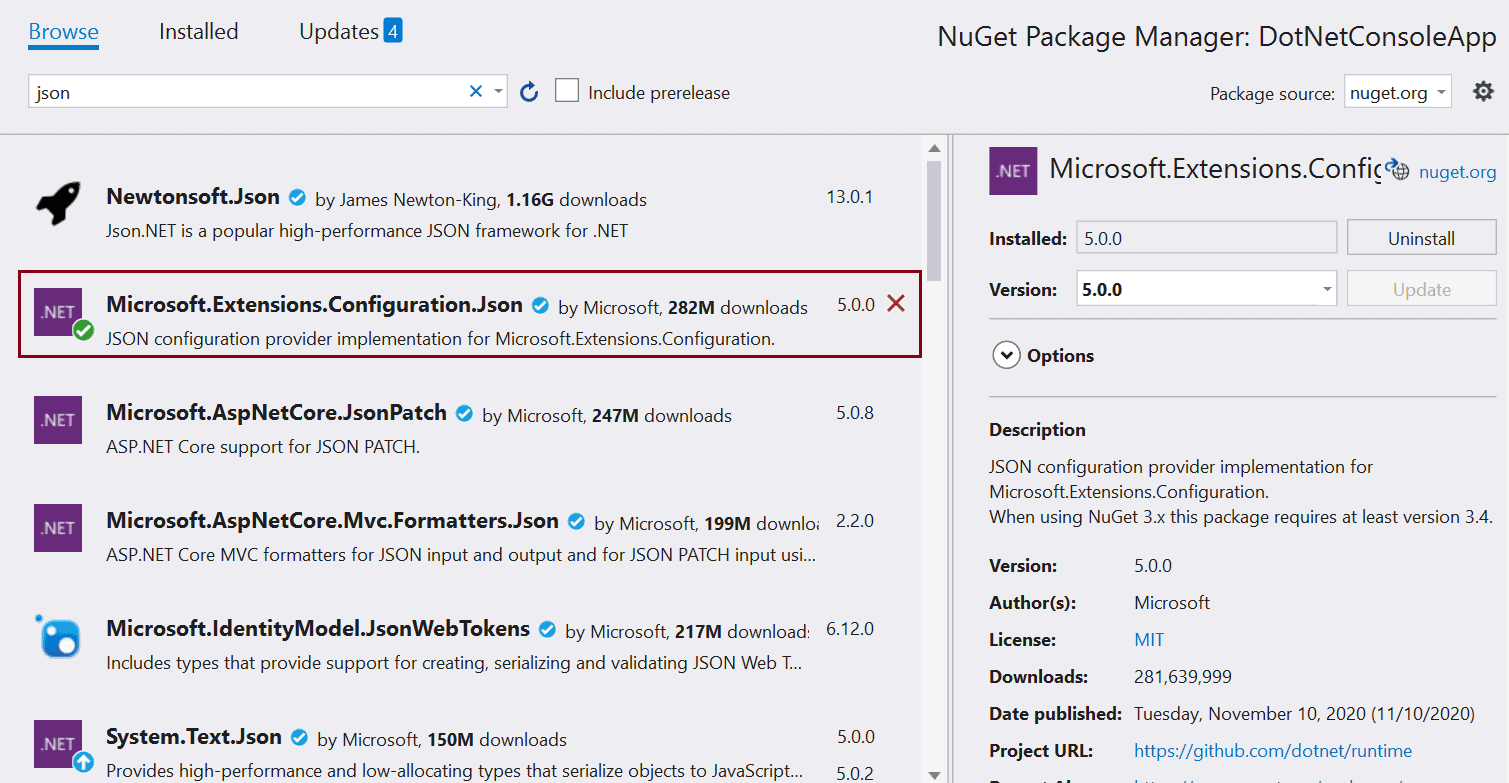
Convert an Object to a Minified JSON String
The following example shows the conversion of an object to a minified JSON string using the JsonSerializer
class.Serialize method:
using System;
using System.Text.Json;
namespace ObjectToJSONConversion
{
public class Department
{
public int DeptId { get; set; }
public string DepartmentName { get; set; }
}
class Program
{
public static void Main()
{
Department dept= new Department() { DeptId = 101, DepartmentName= "IT" };
string strJson = JsonSerializer.Serialize<Department>(dept);
Console.WriteLine(strJson);
}
}
}
{"DeptId":101,"DepartmentName":"IT"}
As you can see, by default, the JSON string is minified in the above output.
Convert an Object to a Formatted JSON String
The following example shows the conversion of an object to the formatted JSON string:
using System;
using System.Text.Json;
namespace ObjectToJSONConversion
{
public class Department
{
public int DeptId { get; set; }
public string DepartmentName { get; set; }
}
class Program
{
public static void Main()
{
Department dept= new Department() { DeptId = 101, DepartmentName= "IT" };
var opt = new JsonSerializerOptions(){ WriteIndented=true };
string strJson = JsonSerializer.Serialize<Department>(dept, opt);
Console.WriteLine(strJson);
}
}
}
{
"DeptId": 101,
"DepartmentName": "IT"
}
In the above example, we specified an option with WriteIndented=true
as a parameter in the Serialize()
method.
This will return a formatted string with indentation.
Convert a List to a JSON String
The following converts a list collection of objects to JSON array.
using System;
using System.Collections.Generic;
using System.Text.Json;
namespace ObjectToJSONConversion
{
public class Department
{
public int DeptId { get; set; }
public string DepartmentName { get; set; }
}
class Program
{
public static void Main()
{
var deptList = new List<Department>(){
new Department() { DeptId = 101, DepartmentName= "IT" },
new Department() { DeptId = 102, DepartmentName= "Accounts" }
};
var opt = new JsonSerializerOptions(){ WriteIndented=true };
string strJson = JsonSerializer.Serialize<IList<Department>>(deptList, opt);
Console.WriteLine(strJson);
}
}
[
{
"DeptId": 101,
"DepartmentName": "IT"
},
{
"DeptId": 102,
"DepartmentName": "Accounts"
}
]
Convert an Object to a UTF-8 String
Serialization to an utf-8 byte array is a bit faster than the string method. This is because the bytes of utf-8 is not required to convert to strings of utf-16.
The following example shows the conversion of an object to a minified JSON string using JsonSerializer.SerializeToUtf8Bytes
method
using System;
using System.Text.Json;
namespace ObjectToJSONConversion
{
public class Department
{
public int DeptId { get; set; }
public string DepartmentName { get; set; }
}
class Program
{
public static void Main()
{
Department dept= new Department() { DeptId = 101, DepartmentName= "IT" };
byte[] utf8bytesJson = JsonSerializer.SerializeToUtf8Bytes(dept);
string strJson = System.Text.Encoding.UTF8.GetString(utf8bytesJson);
Console.WriteLine(strJson);
}
}
}
{"DeptId":101,"DepartmentName":"IT"}
Thus, you can convert C# object to JSON in different ways for different versions using JsonConvert.Serialize()
method in .NET 4.x and .NET 5.
- How to get the sizeof a datatype in C#?
- Difference between String and StringBuilder in C#
- Static vs Singleton in C#
- Difference between == and Equals() Method in C#
- Asynchronous programming with async, await, Task in C#
- How to loop through an enum in C#?
- Generate Random Numbers in C#
- Difference between Two Dates in C#
- Convert int to enum in C#
- BigInteger Data Type in C#
- Convert String to Enum in C#
- Convert JSON String to Object in C#
- DateTime Formats in C#
- How to convert date object to string in C#?
- Compare strings in C#
- How to count elements in C# array?
- Difference between String and string in C#.
- How to get a comma separated string from an array in C#?
- Boxing and Unboxing in C#
- How to convert string to int in C#?