How to use web.config customErrors in ASP.NET MVC
The <customErrors>
element under system.web
in web.config is used to configure error code to a custom page. It can be used to configure custom pages for any error code 4xx or 5xx. However, it cannot be used to log exception or perform any other action on exception.
ASP.NET MVC application displays the flowing screen (Yellow Screen of Death) by default when an exception occurs that shows the error information if you are running it from the localhost.
When you create an MVC application in Visual Studio, it does not implement any exception handling technique out of the box. It will display an error page when an exception occurred.
For example, consider the following action method that throws an exception.
namespace ExceptionHandlingDemo.Controllers
{
public class HomeController : Controller
{
public ActionResult Contact()
{
string msg = null;
ViewBag.Message = msg.Length; // this will throw an exception
return View();
}
}
Now, navigate to /home/contact
in the browser, and you will see the following yellow page (also known as the Yellow Screen of Death) that shows exception details such as exception type, line number and file name where the exception occurred, and stack trace.
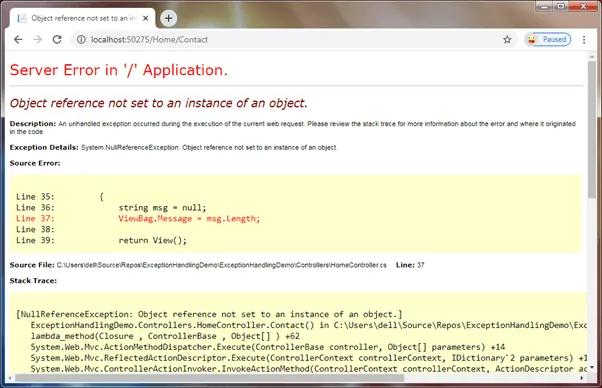
All the unhandled exceptions should be handled and display a meaningful message to the users instead of error information. We can render or redirect to a custom page using <customErrors>
section of web.config file.
The first step is to enable customErrors using its mode attribute that can have one of the following three values:
On: Specifies that custom errors are enabled. If no defaultRedirect is specified, users see a generic error page e.g. Error.cshtml in ASP.NET MVC application.
Off: Specifies that custom errors are disabled. This displays detailed errors.
RemoteOnly: Specifies that custom errors are shown only to remote clients, and ASP.NET errors are shown to the localhost. This is the default.
We will use Mode=On to test it on the localhost. Ideally, we should use RemoteOnly.
<system.web>
<customErrors mode="On"></customErrors>
</system.web>
You also need to add HandleErrorAttribute
filter in the FilterConfig.cs
file.
public class FilterConfig
{
public static void RegisterGlobalFilters(GlobalFilterCollection filters)
{
filters.Add(new HandleErrorAttribute());
}
}
After enabling the customErrors mode to On, an ASP.NET MVC application will show the default custom error page, as shown below.
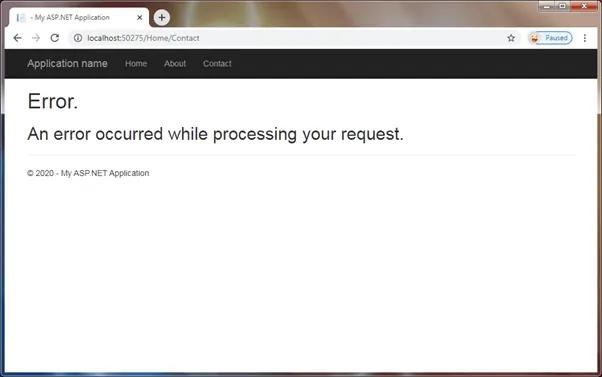
Surprised! How it shows the above page, and from where?
Well! It has rendered Error.cshtml view from the Shared folder. The HandleErrorAttribute filter set this default error view in ASP.NET MVC.
Check the status code of the response in a developer console by pressing F12 (in Chrome) and refresh the page again. You will see it has responded with the status code 500.
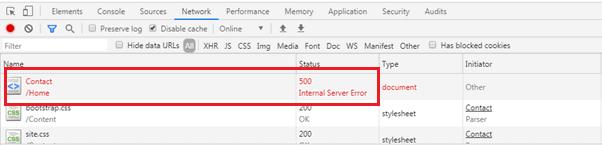
This setting will only show a custom page for 500 level errors, but not for other error codes.
Use the defaultRedirect
to specify where an http request should be redirect by default if an error occurs. You can specify the name of a webform, an HTML, or an action method. This will redirect to this page on any error code, not just 500.
You have to remove HttpErrorHandler
filter if you use the defaultRedirect
attribute. Otherwise, it will redirect to the default Error.cshtml page, not the configured one.
For example, the following configuration will display a custom webform Error.aspx page on any 5xx or 4xx exceptions.
<system.web>
<customErrors mode="On" defaultRedirect="Error.aspx" >
</customErrors>
</system.web>
The following configuration will display a custom HTML page Error.html on any 5xx or 4xx exception.
<system.web>
<customErrors mode="On" defaultRedirect="Error.html" >
</customErrors>
</system.web>
You can also redirect to the controller's action method when an exception occurred. The following setting will redirect to the Index action method of the ErrorController in MVC application.
<system.web>
<customErrors mode="On" defaultRedirect="/error" >
</customErrors>
</system.web>
The above setting will display the following result in the browser.
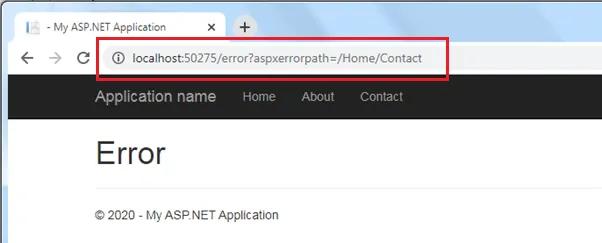
Notice that all the custom error pages returned with the status code 200, and an URL includes ?aspxerrorpath=
query string that points the original requested page or action method.
You can also set different action methods or pages for different error codes using child <error>
elements. The following sets different action methods for different error codes.
<customErrors mode="On" defaultRedirect="/error" >
<error statusCode="400" redirect="/error/badrequest" />
<error statusCode="404" redirect="/error/notfound" />
<error statusCode="500" redirect="/error/internalerror" />
</customErrors>
Of course, you have to create all the above action methods and their views. The above settings will display the following result when navigating to a non-existent page /home/test
.
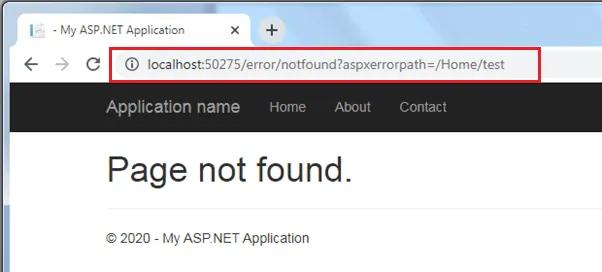
As you have seen, URLs include a query string ?aspxerrorpath
query string when a custom error page displays. You can preserve the original URL using the redirectMode attribute.
redirectMode
Use the redirectMode
attribute if you want to preserve the original URL without ?aspxerrorpath
query string. By default, it has redirectMode set to ResponseRedirect, and that's why the URL gets changed when an exception occurred.
Set the redirectMode
attribute to ResponseRewrite
. This will keep the original URL but still display a custom page. Note that ResponseRewrite is only applicable for .aspx, or .html files, but not for action methods. If you want to set action methods for different status codes, keep the ResponseRedirect
value.
The following sets different HTML files for different status codes and redirectMode
to ResponseRewrite
.
<customErrors mode="On" redirectMode="ResponseRewrite" defaultRedirect="~/500.html" >
<error statusCode="500" redirect="~/500.html" />
<error statusCode="404" redirect="~/404.html" />
<error statusCode="400" redirect="~/400.html" />
</customErrors>
Create 500.html, 404.html, and 400.html files at the root of the application. The above settings will keep the original URL without a query string while showing the inner content of an HTML page with the response status code 200 OK.
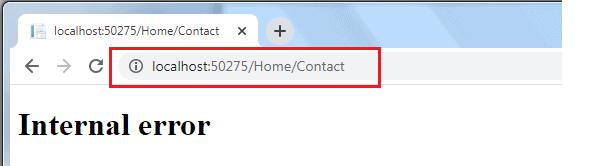
Thus, you can use <customErrors>
section in web.config to display custom pages when exceptions occurred in an ASP.NET webform or MVC applications.
The <customErrors> configuration will always return a response with 200 status code. This is not a good SEO practice for websites. Learn How to display a custom error page and return error code using httpErrors in ASP.NET MVC.