Serve static files from different folder than wwwroot folder in ASP.NET Core
You can configure middleware to serve static files from other folders along with default web root folder wwwroot.
For example, we will server admin.html from the following admin folder and also test.html from wwwroot folder.
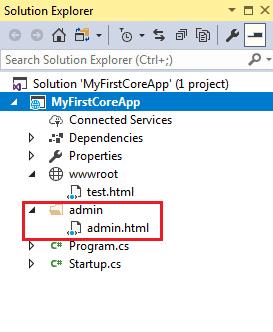
Now, configure the StaticFiles middleware in the Configure() method of Startup class as shown below.
Example: Configure StaticFiles Middleware
public class Startup
{
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IHostingEnvironment env, ILoggerFactory loggerFactory)
{
app.UseStaticFiles();
app.UseStaticFiles(new StaticFileOptions() {
FileProvider = new PhysicalFileProvider(Path.Combine(Directory.GetCurrentDirectory(), "Content")),
RequestPath = new PathString("/Admin")
});
app.Run(async (context) =>
{
await context.Response.WriteAsync("Hello World!");
});
}
}
As you can see, app.UseStaticFiles()
enables default web root folder wwwroot to serve the static files.
app.UseStaticFiles(new StaticFileOptions() {
FileProvider = new PhysicalFileProvider(Path.Combine(Directory.GetCurrentDirectory(), "admin")),
RequestPath = new PathString("/admin")
});
The above code configures Content admin folder to serve static files on the request path /admin. So now, we will be able to execute HTTP request http://localhost:1234/admin/admin.html to display static admin.html page.