Configure Web API
Web API supports code based configuration. It cannot be configured in web.config file. We can configure Web API to customize the behaviour of Web API hosting infrastructure and components such as routes, formatters, filters, DependencyResolver, MessageHandlers, ParamterBindingRules, properties, services etc.
We created a simple Web API project in the Create Web API Project section. Web API project includes default WebApiConfig class in the App_Start folder and also includes Global.asax as shown below.
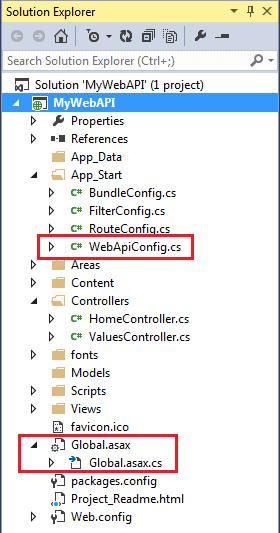
public class WebAPIApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
GlobalConfiguration.Configure(WebApiConfig.Register);
//other configuration
}
}
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
config.MapHttpAttributeRoutes();
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
// configure additional webapi settings here..
}
}
Web API configuration process starts when the application starts. It calls GlobalConfiguration.Configure(WebApiConfig.Register)
in the Application_Start method. The Configure()
method requires the callback method where Web API has been configured in code. By default this is the static WebApiConfig.Register()
method.
As you can see above, WebApiConfig.Register()
method includes a parameter of HttpConfiguration
type which is then used to configure the Web API. The HttpConfiguration
is the main class which includes following properties using which you can override the default behaviour of Web API.
Property | Description |
---|---|
DependencyResolver | Gets or sets the dependency resolver for dependency injection. |
Filters | Gets or sets the filters. |
Formatters | Gets or sets the media-type formatters. |
IncludeErrorDetailPolicy | Gets or sets a value indicating whether error details should be included in error messages. |
MessageHandlers | Gets or sets the message handlers. |
ParameterBindingRules | Gets the collection of rules for how parameters should be bound. |
Properties | Gets the properties associated with this Web API instance. |
Routes | Gets the collection of routes configured for the Web API. |
Services | Gets the Web API services. |
Visit MSDN to learn about all the members of HttpConfiguration.
Learn how to configure Web API routes in the next section.