Web API Routing
In the previous section, we learned that Web API can be configured in WebApiConfig class. Here, we will learn how to configure Web API routes.
Web API routing is similar to ASP.NET MVC Routing. It routes an incoming HTTP request to a particular action method on a Web API controller.
Web API supports two types of routing:
- Convention-based Routing
- Attribute Routing
Convention-based Routing
In the convention-based routing, Web API uses route templates to determine which controller and action method to execute. At least one route template must be added into route table in order to handle various HTTP requests.
When we created Web API project using WebAPI template in the Create Web API Project section, it also added WebApiConfig class in the App_Start folder with default route as shown below.
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
// Enable attribute routing
config.MapHttpAttributeRoutes();
// Add default route using convention-based routing
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
}
}
In the above WebApiConfig.Register() method, config.MapHttpAttributeRoutes()
enables attribute routing which we will learn later in this section. The config.Routes
is a route table or route collection of type HttpRouteCollection. The "DefaultApi" route is added in the route table using MapHttpRoute() extension method. The MapHttpRoute()
extension method internally creates a new instance of IHttpRoute and adds it to an HttpRouteCollection. However, you can create a new route and add it into a collection manually as shown below.
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
config.MapHttpAttributeRoutes();
// define route
IHttpRoute defaultRoute = config.Routes.CreateRoute("api/{controller}/{id}",
new { id = RouteParameter.Optional }, null);
// Add route
config.Routes.Add("DefaultApi", defaultRoute);
}
}
The following table lists parameters of MapHttpRoute() method.
Parameter | Description |
---|---|
name | Name of the route |
routeTemplate | URL pattern of the route |
defaults | An object parameter that includes default route values |
constraints | Regex expression to specify characteristic of route values |
handler | The handler to which the request will be dispatched. |
Now, let's see how Web API handles an incoming http request and sends the response.
The following is a sample HTTP GET request.
GET http://localhost:1234/api/values/ HTTP/1.1 User-Agent: Fiddler Host: localhost: 60464 Content-Type: application/json
Considering the DefaultApi
route configured in the above WebApiConfig
class, the above request will execute Get()
action method of the ValuesController
because HTTP method is a GET and URL is http://localhost:1234/api/values
which matches with DefaultApi
's route template /api/{controller}/{id}
where value of {controller}
will be ValuesController
. Default route has specified id as an optional parameter so if an id is not present in the url then it will be ignored. The request's HTTP method is GET
so it will execute Get()
action method of ValueController
.
If Web API framework does not find matched routes for an incoming request then it will send 404 error response.
The following figure illustrates Web API Routing.
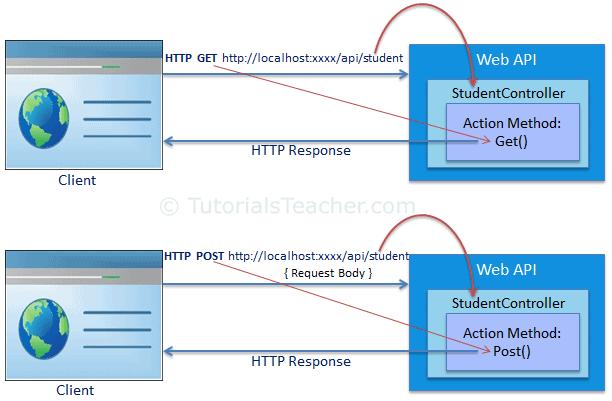
The following table displays which action method and controller will be executed on different incoming requests.
Request URL | Request HTTP Method | Action method | Controller |
---|---|---|---|
http://localhost:1234/api/course | GET | Get() | CourseController |
http://localhost:1234/api/product | POST | Post() | ProductController |
http://localhost:1234/api/teacher | PUT | Put() | TeacherController |
Web API also supports routing same as ASP.NET MVC by including action method name in the URL.
Configure Multiple Routes
We configured a single route above. However, you can configure multiple routes in the Web API using HttpConfiguration object. The following example demonstrates configuring multiple routes.
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
config.MapHttpAttributeRoutes();
// school route
config.Routes.MapHttpRoute(
name: "School",
routeTemplate: "api/myschool/{id}",
defaults: new { controller="school", id = RouteParameter.Optional }
constraints: new { id ="/d+" }
);
// default route
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
}
}
In the above example, School route is configured before DefaultApi route. So any incoming request will be matched with the School route first and if incoming request url does not match with it then only it will be matched with DefaultApi route. For example, request url is http://localhost:1234/api/myschool
is matched with School route template, so it will be handled by SchoolController.
Visit asp.net to learn about routing in detail.
Attribute Routing
Attribute routing is supported in Web API 2. As the name implies, attribute routing uses [Route()] attribute to define routes. The Route
attribute can be applied on any controller or action method.
In order to use attribute routing with Web API, it must be enabled in WebApiConfig by calling config.MapHttpAttributeRoutes()
method.
Consider the following example of attribute routing.
public class StudentController : ApiController
{
[Route("api/student/names")]
public IEnumerable<string> Get()
{
return new string[] { "student1", "student2" };
}
}
In the above example, the Route
attribute defines new route "api/student/names" which will be handled by the Get() action method of StudentController. Thus, an HTTP GET request http://localhost:1234/api/student/names
will return list of student names.
Visit asp.net to learn about attribute routing in detail.