Create Simple Go Program
In this article, let us write the first program Hello World
in Go language using Visual Studio Code editor.
First of all, create a folder "HelloWorld". Now, open visual studio code. From the file menu, open the "HelloWorld" folder we created just now. This is also called as a package in Go.
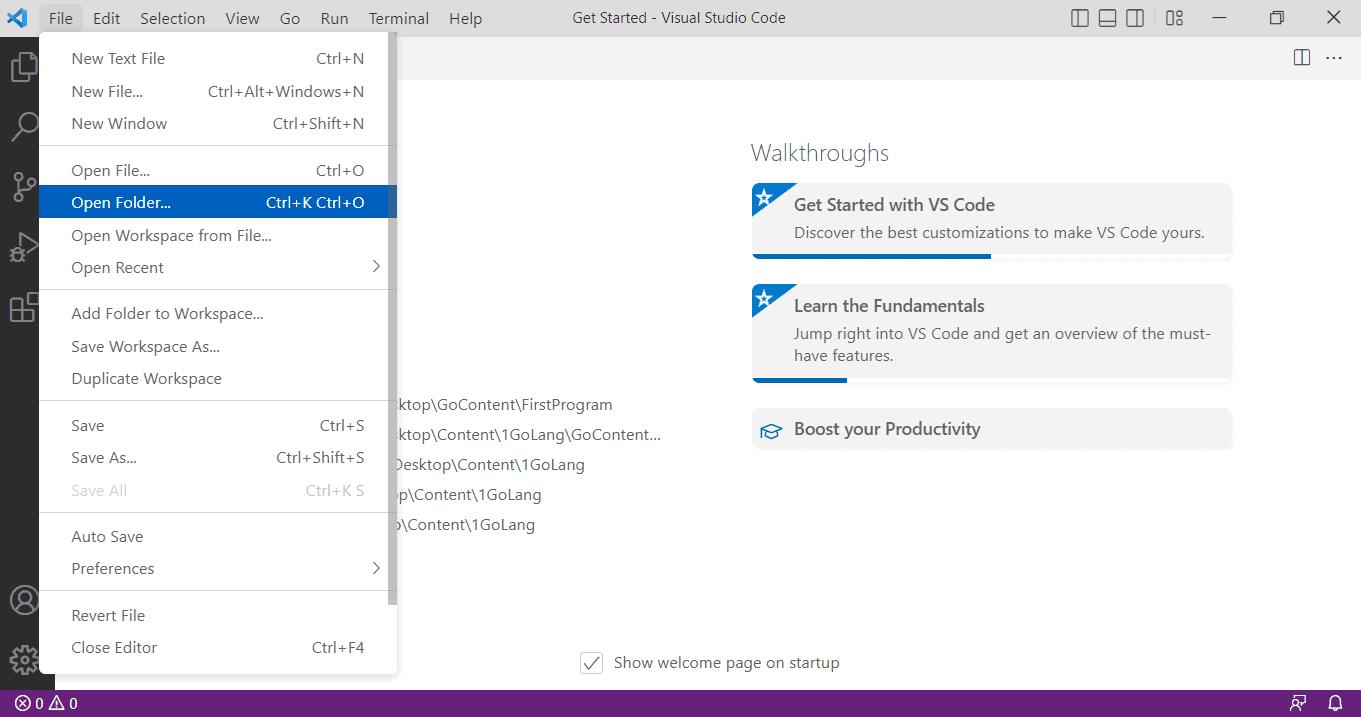
Now, create a new file in VSCode and name it main.go
, as shown below.
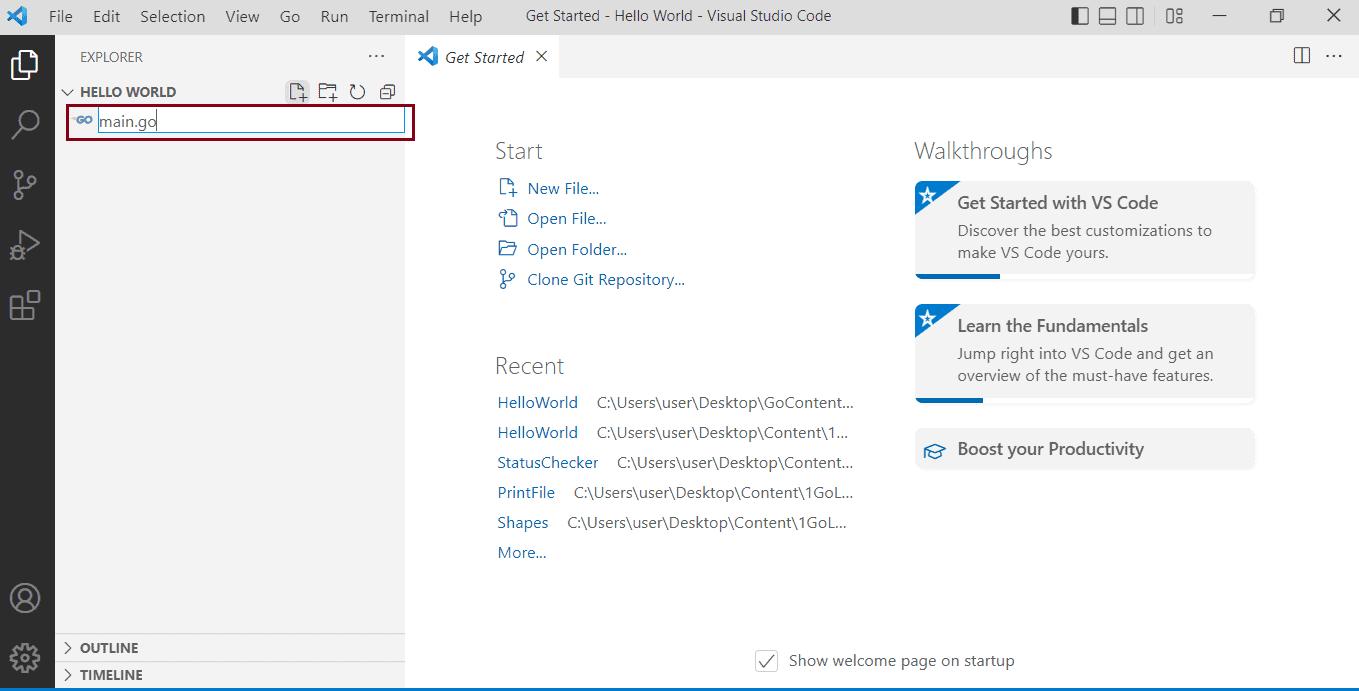
All files in Go language will have the .go
extension. There are two types of programs you can build in Go. One is an executable and the other, a library. Executable programs can be executed directly from the terminal, whereas libraries are packages whose code can be reused and can be included in other libraries or executable programs.
Our HelloWorld program is an executable which can be run independently and the output printed on the terminal.
Every executable program starts with the main
package declaration which tells the Go compiler that the program is an executable.
package main
Next you import the "fmt" package. The fmt
package includes functions for text formatting including printing to the console.
package main
import "fmt"
After the import, write the function named main()
that prints "Hello World" to the console.
package main
import "fmt"
func main () {
fmt.Println("Hello, World!")
}
In the above example, func
is a keyword used to define a function in Go. func main()
defines the function named main. The main()
is the starting point function in Go which does not accept any parameter and does not return any value. The main package must have a main()
function in Go.
The Println()
is the function in the fmt
package that prints the specified value to the terminal/command prompt.
The below image shows the HelloWorld program code in the VSCode editor.
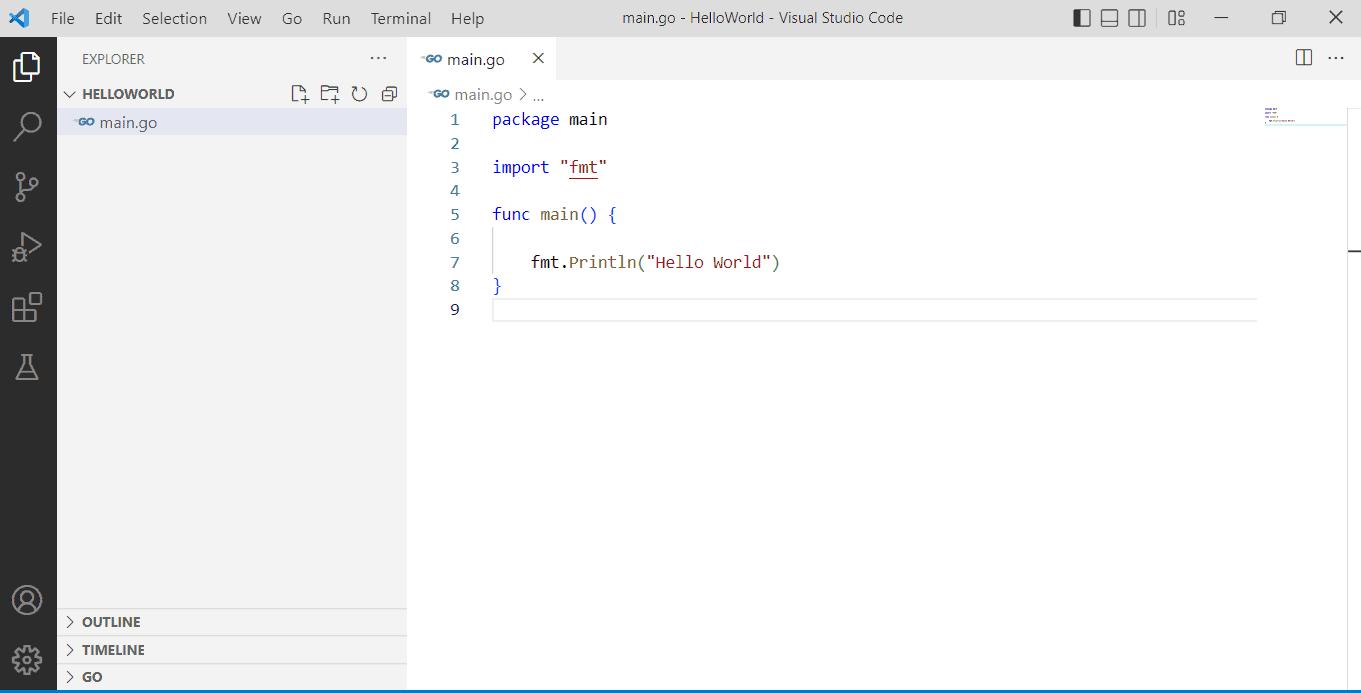
To execute the code, you can open a new Terminal within the VSCode editor. This will automatically navigate to the directory of the package, as shown below.
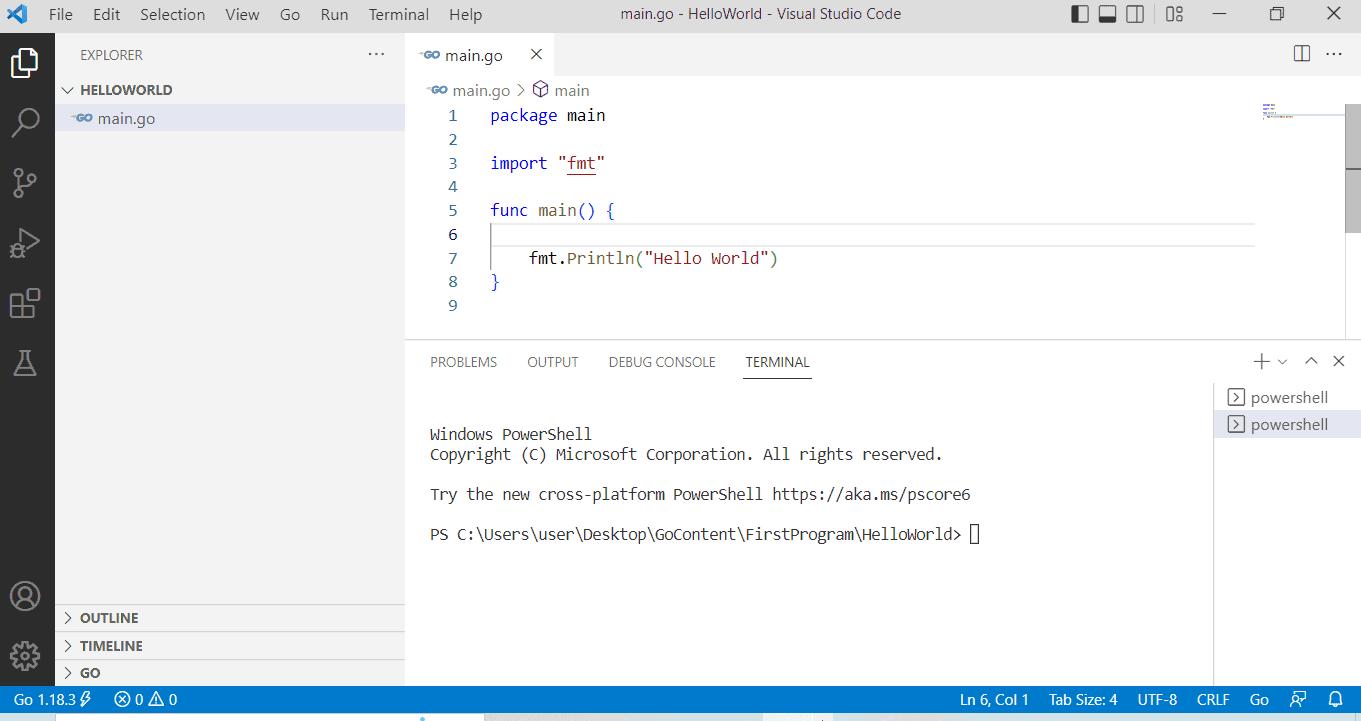
Now, enter the command Go run main.go
to run the program, as shown below.
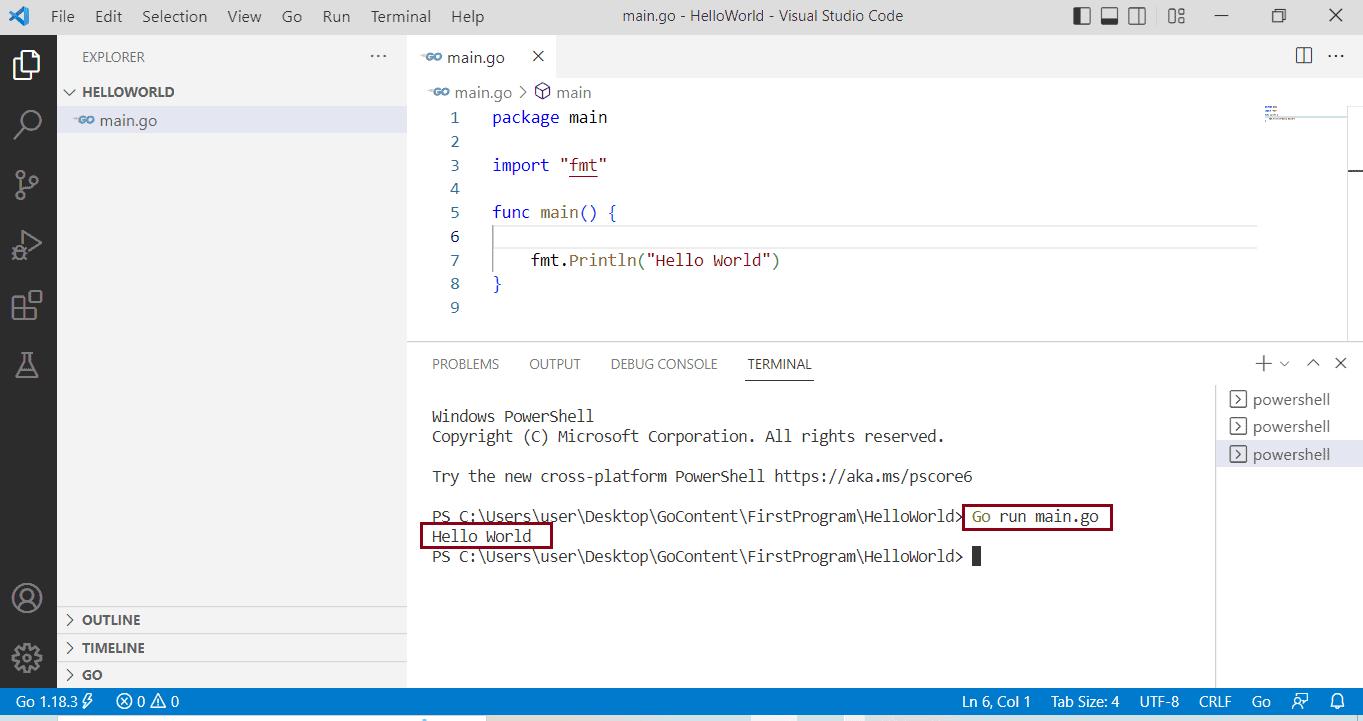
Alternatively, you can open the command prompt in Windows and navigate to the program directory and execute the same command to run the program, as shown below.
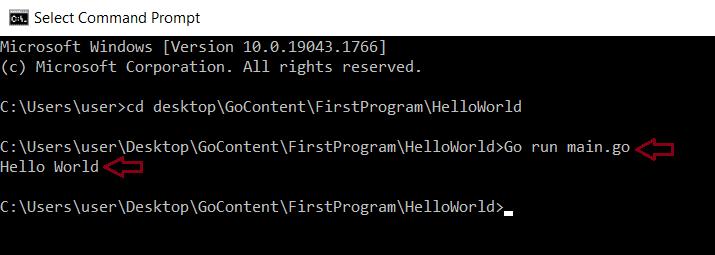
Congrats! You have created and run the first simple Go program using VS Code.