Create Modules in Go
In Go lang, a module is a dependency management feature. Go modules make it easier to maintain various versions and adds flexibility in organizing a project.
A module is a collection of packages stored in a file tree under $GOPATH/pkg
folder with a go.mod
file at its root. This file defines the module's path which is also the import path used for the root directory and its dependency requirements.
The Go command automatically checks and adds dependencies required for imports provided the current directory or the parent directory has a go.mod
fie.
Creating a Go Module
A Go module will have several Go files or packages in addition to two important files in the root, the go.mod
file and go.sum
file. These files are maintained by the Go tool and it is used to track the module's configuration.
Before creating a module, you need to identify a directory where the module will reside. This directory can be anywhere on the computer and need not be in any specific Go directory. You can use an existing directory or create a new one.
Create a Module
Create a new directory called MyProject
. It can be created using an IDE or the command prompt. Use mkdir MyProject
command on windows command prompt, as shown below.
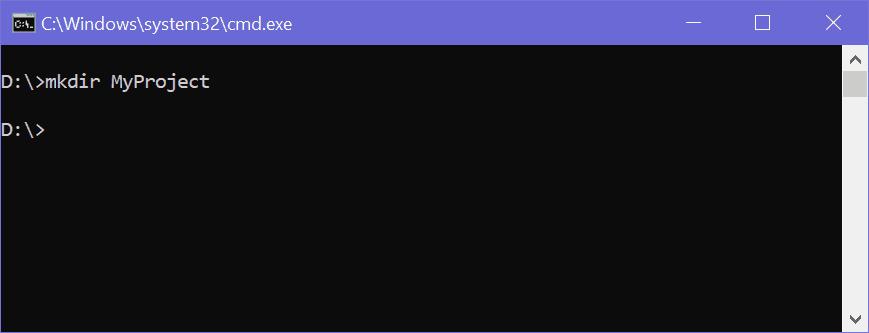
Now, navigate to the MyProject
folder using cd MyProject
command.
Next, create the module directory MyModule
under the MyProject
directory.
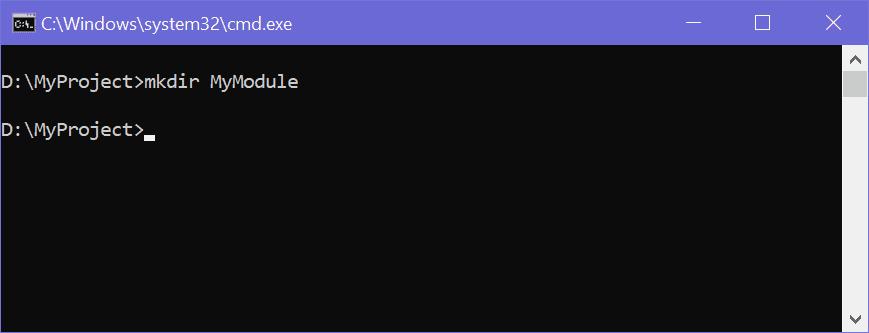
Note: If you are using an IDE like VSCode, you can open the directory and create folders/modules, packages, and files in the IDE.
Once the module is created, create the go.mod
file within the module MyModule
using the following command:
go mod init MyModule
This will create the go.mod
file under the MyModule
folder.
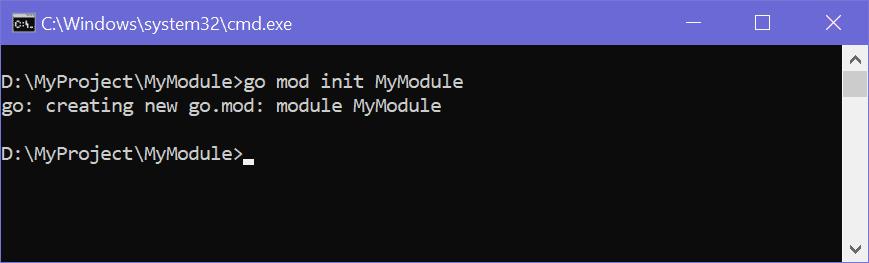
The newly created go.mod file will have the module name and the go version which the module is targeting. This file will expand as more information is added to the module.
Now, you can start adding files to the newly created module. First, create the main.go
file to run the go module. The main.go
file is the starting point of a go program. The name of the file is not important (can have any name) but the main()
function within this file is the entry point for the program. So having the file name as main.go
makes it easier to find the starting point.
A module can contain one or more packages and sub-packages. Entire folder structure will look like below.
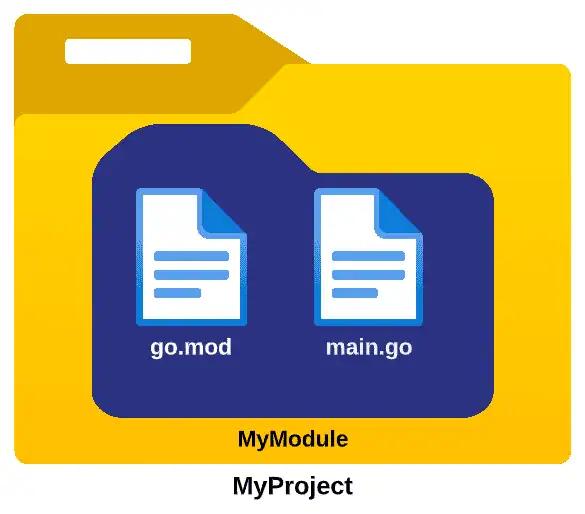
Open the main.go
file and add the following code and save. This prints My first Go module!
as output.
package main
import "fmt"
func main() {
fmt.Println("My first Go module!")
}
Every file in go will start with the package declaration. In the above example, the package is named main. The main word specifies to the go compiler that the package is binary and should be compiled into an executable file. Any other name will compile the file as a library or utility package to be used in other files.
After declaring the package, the import "fmt" line imports the format library into the file using which print statements can be used.
Use the following command to run the go program
go run main.go
</code></pre>
</div>
<div className="card-footer example-footer"></div>
</div>
<p>This will print <code>My first Go module!</code> output on console.</p>
<ResponsiveImage src="/images/go/module5.webp" alt="" lazyLoad={true} />
<VideoAd />
<h2>Adding a Module to another Module as a Dependency</h2>
<p>A remote module can be accessed from another module by importing it into the calling module. In the following simple example, create two modules: the sales module and the products module. The products module will call the sales module.</p>
<p>Create a directory named sales. Create the module with the import path <code>Sample.com/sales</code></p>
<p>Create a file <code>sales.go</code> in the sales directory with the following code:</p>
<div className="card code-panel-without-title">
<div className="panel-body">
<pre className="language-go"><code>package sales
function total(cost, profit int) int {
return cost + profit
}
</code></pre>
</div>
<div className="card-footer example-footer"></div>
</div>
<p>Next, create the products directory in the same path as the sales directory. Create the products module. Next, modify the <code>go.mod</code> file to import the sales module into the products module.</p>
<p>Now, create a <code>products.go</code> file which imports the sales module and uses its total function.</p>
<CodeExample
title="Example: products.go"
language="language-go"
>
{`package main
import (
"fmt"
"sample.com/sales" //import the sales module
)
func main() {
fmt.Println(sales.total(200, 40))
}`}
</CodeExample>
<p>Run the products.go file.</p>
<div className="card code-panel-without-title">
<div className="panel-body">
<pre className="language-go"><code>go run products.go
The program can access the total function available in the sales module and returns 240 as output.
A specific version of the Go module can be used as Go modules are distributed from a version control repository and they can use version control features like tags, branches, and commits. You can specify the version of the module that will be used in the dependency with @ symbol at the end of the module path as shown below.
go get sample.com/sales@latest
Visit go modules for more information.