MongoDB Documents: Document, Array, Embedded Document
In the RDBMS database, a table can have multiple rows and columns. Similarly in MongoDB, a collection can have multiple documents which are equivalent to the rows. Each document has multiple "fields" which are equivalent to the columns. So in simple terms, each MongoDB document is a record and a collection is a table that can store multiple documents.
The following is an example of JSON based document.
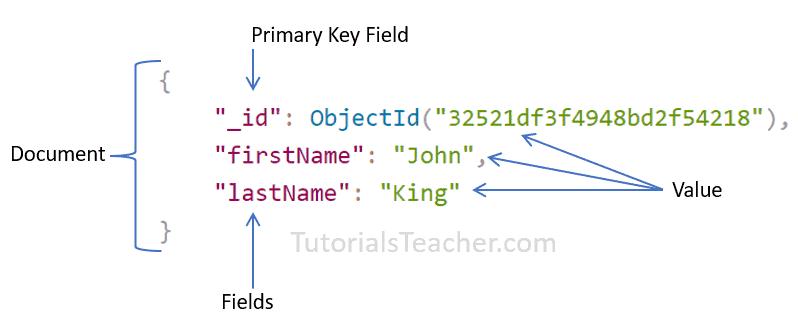
In the above example, a document is contained within the curly braces. It contains multiple fields in "field":"value"
format. Above, "_id"
, "firstName"
, and "lastName"
are field names with their respective values after a colon :
. Fields are separated by a comma. A single collection can have multiple such documents separated by a comma.
The following chart to understand the relation between database, collections, and documents.
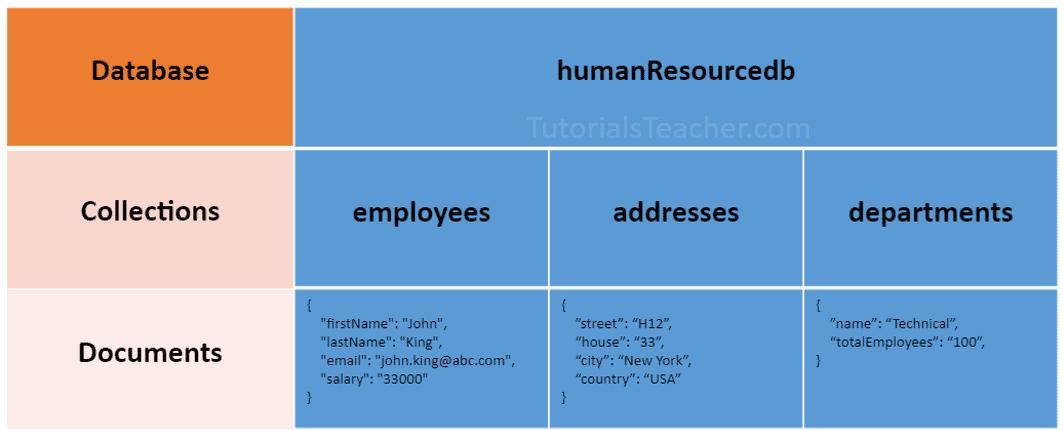
The following is an example of a document that contains an array and an embedded document.
{
"_id": ObjectId("32521df3f4948bd2f54218"),
"firstName": "John",
"lastName": "King",
"email": "[email protected]",
"salary": "33000",
"DoB": new Date('Mar 24, 2011'),
"skills": [ "Angular", "React", "MongoDB" ],
"address": {
"street":"Upper Street",
"house":"No 1",
"city":"New York",
"country":"USA"
}
}
MongoDB document stores data in JSON format. In the above document, "firstName", "lastName", "email", and "salary" are the fields (like columns of a table in RDBMS) with their corresponding values (e.g value of a column in a row). Consider "_id"
field as a primary key field that stores a unique ObjectId."skills"
is an array and "address"
holds another JSON document.
The field names can be specified without surrounding quotation marks, as shown below.
{
_id: ObjectId("32521df3f4948bd2f54218"),
firstName: "John",
lastName: "King",
email: "[email protected]",
salary: "33000",
DoB: new Date('Mar 24, 2011'),
skills: [ "Angular", "React", "MongoDB" ],
address: {
street:"Upper Street",
house:"No 1",
city:"New York",
country:"USA"
}
}
MongoDB stores data in key-value pairs as a BSON document. BSON is a binary representation of a JSON document that supports more data types than JSON. MongoDB drivers convert JSON document to BSON data.

Important Points:
- MongoDB reserves
_id
name for use as a unique primary key field that holds ObjectId type. However, you are free to give any name you like with any data type other than the array. - A document field name cannot be
null
but the value can be. - Most MongoDB documents cannot have duplicate field names. However, it depends on the driver you use to store a document in your application.
- A document fields can be without quotation marks
" "
if it does not contain spaces, e.g.{ name: "Steve"}
,{ "first name": "Steve"}
are valid fields. - Use the dot notation to access array elements or embedded documents.
- MongoDB supports maximum document size of 16mb. Use GridFS to store more than 16 MB document.
- Fields in a BSON document are ordered. It means fields order is important while comparing two documents, e.g.
{x: 1, y: 2}
is not equal to{y: 2, x: 1}
- MogoDB keeps the order of the fields except
_id
field which is always the first field. - MongoDB collection can store documents with different fields. It does not enforce any schema.
Embedded Documents:
A document in MongoDB can have fields that hold another document. It is also called nested documents.
The following is an embedded document where the department
and address
field contains another document.
{
_id: ObjectId("32521df3f4948bd2f54218"),
firstName: "John",
lastName: "King",
department: {
_id: ObjectId("55214df3f4948bd2f8753"),
name:"Finance"
},
address: {
phone: { type: "Home", number: "111-000-000" }
}
}
In the above embedded document, notice that the address
field contains the phone
field which holds a second level document.
- An embedded document can contain upto 100 levels of nesting.
- Supports a maximum size of 16 mb.
- Embedded documents can be accessed using dot notation
embedded-document.fieldname
, e.g. access phone number usingaddress.phone.number
.
Array
A field in a document can hold array. Arrays can hold any type of data or embedded documents.
Array elements in a document can be accessed using dot notation with the zero-based index position and enclose in quotes.
{
_id: ObjectId("32521df3f4948bd2f54218"),
firstName: "John",
lastName: "King",
email: "[email protected]",
skills: [ "Angular", "React", "MongoDB" ],
}
The above document contains the skills
field that holds an array of strings. To specify or access the second element in the skills
array, use skills.1
.