Area in ASP.NET MVC
Here, you will learn what an area in ASP.NET MVC application is and how to create it.
The large ASP.NET MVC application includes many controllers, views, and model classes. So it can be difficult to maintain it with the default ASP.NET MVC project structure. ASP.NET MVC introduced a new feature called Area for this. Area allows us to partition the large application into smaller units where each unit contains a separate MVC folder structure, same as the default MVC folder structure. For example, a large enterprise application may have different modules like admin, finance, HR, marketing, etc. So an Area can contain a separate MVC folder structure for all these modules, as shown below.
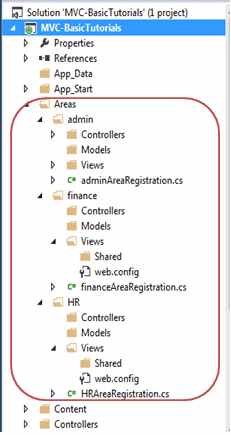
Creating an Area
You can create an area by right-clicking on the project in the solution explorer -> Add
-> Area..
, as shown below.
Enter the name of an area in the Add Area
dialogue box and click on the Add button.

This will add an admin
folder under the Area
folder, as shown below.

As you can see, each area includes the AreaRegistration
class.
The following is adminAreaRegistration
class created with admin area.
public class adminAreaRegistration : AreaRegistration
{
public override string AreaName
{
get
{
return "admin";
}
}
public override void RegisterArea(AreaRegistrationContext context)
{
context.MapRoute(
"admin_default",
"admin/{controller}/{action}/{id}",
new { action = "Index", id = UrlParameter.Optional }
);
}
}
The AreaRegistration
class overrides the RegisterArea
method to map the routes for the area. In the above example, any URL that starts with the admin will be handled by the controllers included in the admin folder structure under the Area
folder.
For example, http://localhost/admin/profile
will be handled by the profile controller included in the Areas/admin/controller/ProfileController
folder.
Finally, all the areas must be registered in the Application_Start
event in Global.asax.cs
as AreaRegistration.RegisterAllAreas();
.
So in this way, you can create and maintain multiple areas for the large application.