Bind Query String to an Action Method Parameters in MVC
Here, you will learn about to bind a model object to an action method parameters in the ASP.NET MVC application.
The model binding refers to converting the HTTP request data (from the query string or form collection) to an action method parameters. These parameters can be of primitive type or complex type.
Binding to Primitive Type
The HTTP GET request embeds data into a query string.
MVC framework automatically converts a query string to the action method parameters provided their names are matching.
For example, the query string id
in the following GET request would automatically be mapped to the Edit()
action method's id
parameter.


You can also have multiple parameters in the action method with different data types. Query string values will be converted into parameters based on the matching names.
For example, the query string parameters of an HTTP request http://localhost/Student/Edit?id=1&name=John
would map to id
and name
parameters of the following Edit()
action method.
public ActionResult Edit(int id, string name)
{
// do something here
return View();
}
Binding to Complex Type
Model binding also works on complex types. It will automatically convert the input fields data on the view to the properties of a complex type parameter of an action method in HttpPost request if the properties' names match with the fields on the view.
public class Student
{
public int StudentId { get; set; }
public string StudentName { get; set; }
public int Age { get; set; }
public Standard standard { get; set; }
}
public class Standard
{
public int StandardId { get; set; }
public string StandardName { get; set; }
}
Now, you can create an action method which includes the Student type parameter. In the following example, Edit action method (HttpPost) includes Student type parameter.
[HttpPost]
public ActionResult Edit(Student std)
{
var id = std.StudentId;
var name = std.StudentName;
var age = std.Age;
var standardName = std.standard.StandardName;
//update database here..
return RedirectToAction("Index");
}
Thus, the MVC framework will automatically map Form collection values to the Student type parameter when the form submits an HTTP POST request to the Edit()
action method, as shown below.
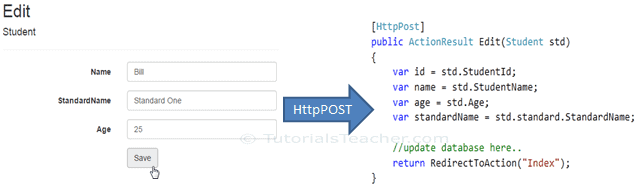
So thus, it automatically binds form fields to the complex type parameter of action method.
FormCollection
You can also include the FormCollection
type parameter in the action method instead of a complex type to retrieve all the values from view form fields, as shown below.

Bind Attribute
ASP.NET MVC framework also enables you to specify which properties of a model class you want to bind.
The [Bind]
attribute will let you specify the exact properties of a model should include or exclude in binding.
In the following example, the Edit()
action method will only bind StudentId
and StudentName
properties of the Student
model class.
[HttpPost]
public ActionResult Edit([Bind(Include = "StudentId, StudentName")] Student std)
{
var name = std.StudentName;
//write code to update student
return RedirectToAction("Index");
}
You can also exclude the properties, as shown below.
[HttpPost]
public ActionResult Edit([Bind(Exclude = "Age")] Student std)
{
var name = std.StudentName;
//write code to update student
return RedirectToAction("Index");
}
The Bind attribute will improve the performance by only bind properties that you needed.
Model Binding Process
As you have seen, that the ASP.NET MVC framework automatically converts request values into a primitive or complex type object. Model binding is a two-step process. First, it collects values from the incoming HTTP request, and second, it populates primitive type or a complex type with these values.
Value providers are responsible for collecting values from requests, and Model Binders are responsible for populating values.
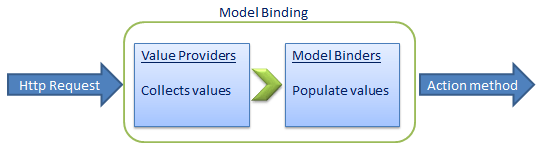
Default value provider collection evaluates values from the following sources:
- Previously bound action parameters, when the action is a child action
- Form fields (Request.Form)
- The property values in the JSON Request body (Request.InputStream), but only when the request is an AJAX request
- Route data (RouteData.Values)
- Querystring parameters (Request.QueryString)
- Posted files (Request.Files)
MVC includes DefaultModelBinder class which effectively binds most of the model types.