Debug Node.js Application
In this section, you will learn ways to debug Node.js application.
You can debug Node.js application using various tools including following:
- Core Node.js debugger
- Node Inspector
- Built-in debugger in IDEs
Core Node.js Debugger
Node.js provides built-in non-graphic debugging tool that can be used on all platforms. It provides different commands for debugging Node.js application.
Consider the following simple Node.js application contained in app.js file.
var fs = require('fs');
fs.readFile('test.txt', 'utf8', function (err, data) {
debugger;
if (err) throw err;
console.log(data);
});
Write debugger in your JavaScript code where you want debugger to stop. For example, we want to check the "data" parameter in the above example. So, write debugger; inside callback function as above.
Now, to debug the above application, run the following command.
node debug app.jsThe above command starts the debugger and stops at the first line as shown below.
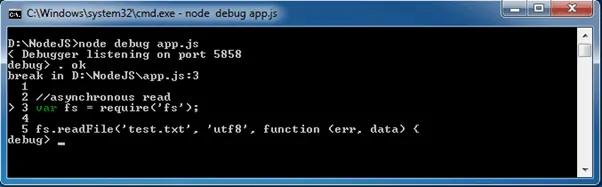
As you can see in the above figure, > symbol indicates the current debugging statement.
Use next to move on the next statement.
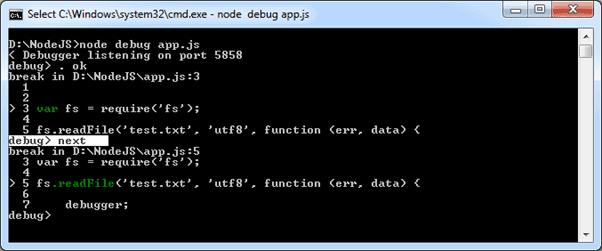
In the above figure, next command will set the debugger on the next line. The > is now pointing to next statement.
Use cont to stop the execution at next "debugger", if any.
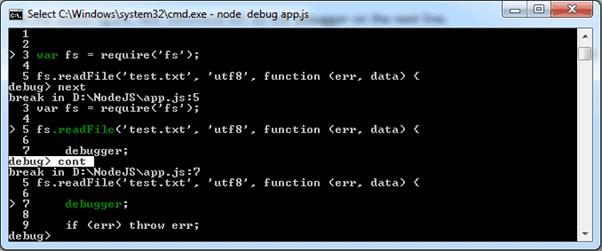
In the above figure, you can see that cont command stops at the "debugger".
Use watch('expression') command to add the variable or expression whose value you want to check. For example, to check the value of data variable in the above example, add data into watch expression as shown below.
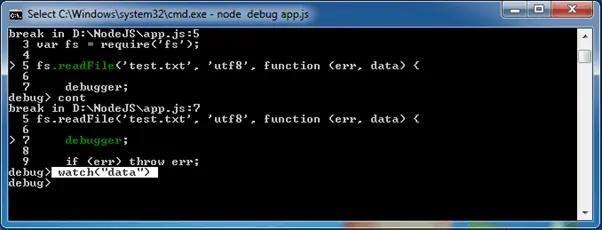
Now, write watchers command to check the value of all the variables added into watch().
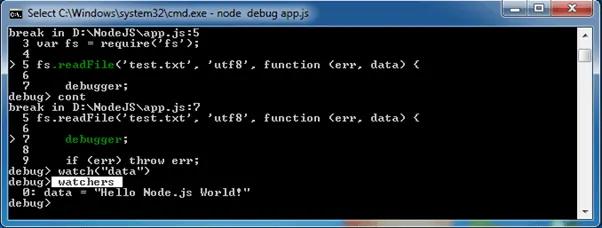
The following table lists important debugging commands:
Command | Description |
---|---|
next | Stop at the next statement. |
cont | Continue execute and stop at the debugger statement if any. |
step | Step in function. |
out | Step out of function. |
watch | Add the expression or variable into watch. |
watcher | See the value of all expressions and variables added into watch. |
Pause | Pause running code. |
Thus, you can use built-in Node.js debugger to debug your Node.js application. Visit Node.js official documentation to know all the Node.js debugging commands or write "help" in debug mode in the Node.js console (REPL).
Learn how to use Node Inspector to debug Node.js application in the next section.