Vash Template Engine
In this section, you will learn about Vash template engine and how to use it in Node.js using Express.js.
Vash is a template view engine that uses Razor Syntax. So, this template engine will look familiar to people who have experience in ASP.Net MVC.
Install Vash using NPM as below.
npm install vash --saveNow, let's see how to render simple vash template using Express.js.
First of all, create index.vash file under views folder and write the following HTML content.
<!DOCTYPE html>
<html>
<head>
<title>@model.title</title>
<meta property="og:title" content="@model.title" />
</head>
<body>
<p>@model.content</p>
</body>
</html>
In the above vash template, the model object is @model and properties are title and content. @model in razor syntax represents an object which will be supplied from the server while rendering this template. So, the above template expects an object with two properties: title and content.
Now, create server.js and write following express.js to render the above vash template.
var express = require('express');
var app = express();
app.set("view engine","vash")
app.get('/', function (req, res) {
res.render('index', { title: 'Vash Template Demo',
content:'This is dummy paragraph.'});
});
var server = app.listen(5000, function () {
console.log('Node server is running..');
});
As you can see, we have specified an object with title and content properties in the res.render() method while rendering index.vash. Now, run the above application using node server.js
command and point your browser to http://localhost:5000 and you will get the following result.
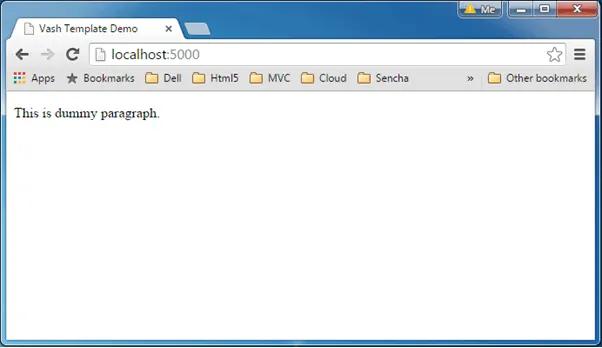
Layout Page
Layout page includes static part of your application which remains the same throughtout your application e.g. header, footer etc. It provides a mechanism to add dynamic content along with the static content.
The vash engine allows you to create a layout page. The following is a simple layout.vash page.
<!DOCTYPE html>
<html>
<head>
<title>@model.title</title>
<meta property="og:title" content="@model.title" />
</head>
<body>
@html.block('body')
</body>
</html>
In the above layout page, @html.block('body') defines the block called "body". So, any vash template can now be injected inside this body block. For example, the following index.vash template injects some HTML into body block of the above layout.vash.
@html.extend('layout',function(model){
@html.block('body',function(model){
<h1>@model.title</h1>
<p>@model.content</p>
});
});
Now, render above vash layout using Express.js as shown below.
var express = require('express');
var app = express();
app.set("view engine","vash")
app.get('/', function (req, res) {
res.render('index', { title: 'Vash Template Demo',
content:'This is dummy paragraph.'});
});
var server = app.listen(5000, function () {
console.log('Server is running..');
});
Now, run the above application using node server.js
command and point your browser to http://localhost:5000 and you will get the following result.
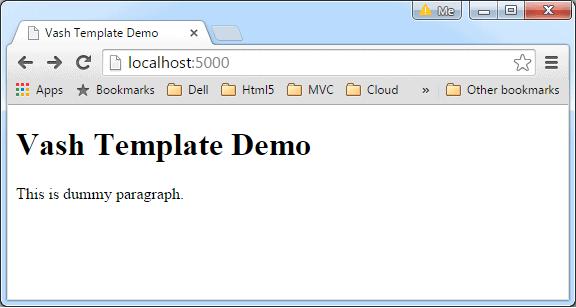
Visit Github to learn vash template syntax in detail.