What is Sass?
Sass (which stands for Syntactically Awesome Style Sheets) is an extension to CSS. It doesn't really change what CSS can do, you won't suddenly be able to use Adobe Photoshop blend modes or anything-but it makes writing CSS a whole lot easier.
Saas includes various features such as variables, nested rules, mixins, inline imports, built-in functions to manipulate color and other values, all with a fully CSS-compatible syntax.
Official website: http://sass-lang.com
Why Sass?
Cascading Style Sheets (CSS) have come a long way since they were first adopted by the W3C in 1996. The days when web developers had to slice up images and use unmaintainable nested tables for layout are long gone (and good riddance). But CSS3, wonderful as it is for those of us who remember the bad old days of limited functionality and spotty implementations isn't quite there yet.
Any non-trivial website still requires repetitive code and multiple rule declarations to support multiple browsers. Let me give you an example: Your client has chosen a simple color scheme for the site, with a primary color, and two accents:
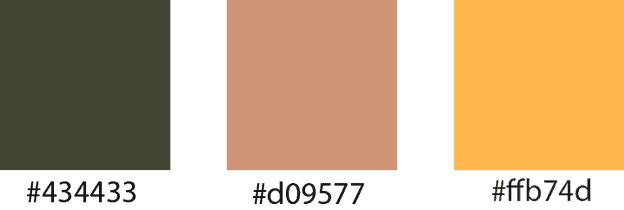
This is basic stuff, right? But how many times do you need to type those numbers? You'll need at least one of them in pretty much every CSS rule you write. (Remember, every time you type something, you have another chance to make a mistake.)
And then you'll probably need to figure out variations on the values. Quick, what's the hex code for the color that's 20% lighter than #434433? You'll probably need it for disabled menu items. And when the designer decides the disabled value should be 15% instead of 20%, you'll need to do the calculation again, and change it every place it's used. CSS3 may be lightyears more functional than CCS1, but that's just ugly, tedious, and more high-maintenance than a pop star on a vegan diet.
Instead of typing #434433 a bazillion times, and changing #76785A to #696B50 when the disabled value percentage changes, you can write this:
/*define a variable for the primary color*/
$primary : #434433;
/*use the lighten() function to determine the disabled value, and define it as a variable*/
$disabled: lighten($primary, 20%);
.menu-item {
color: $primary; /* use primary variable */
.disabled-menu-item {
color: $disabled;/* use disabled variable */
}
Now if the primary color changes, you only change it in one place. If the designer decides to use 15% instead of 20% for the disabled value, you don't have to re-calculate it and you only change it in one place. Even better, because of the way Sass works (we'll talk about that in the next section), you can declare all your variables in one file (by convention, it's a file called _variables.scss) and @include them in every style sheet you write without incurring an extra http call. How sweet is that?
How Sass Works?
You can't send Sass code directly to the browser; it won't know what to do with it. Instead, you need to use the Sass pre-processor to translate the Sass code into standard CSS, a process known as transpiling. Transpilation is very much like compilation, but instead of translating from human-readable source code to machine-readable object code, it translates from one human-readable language to another, in this case from Sass to CSS. You might be familiar with another transpiler used in web development, Babel (), which translates ES2015 into JavaScript that is compatible with downstream browsers. Different languages, same process.
Don't worry about exactly what's going on during the transpilation process. It's one of the (very few) things in our profession that "just works". All you need to understand is that you give the transpiler some Sass code and you get some CSS code back.
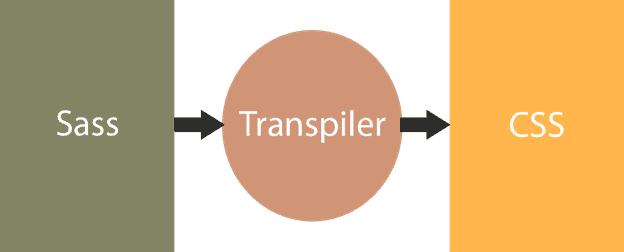
Transpiling from Sass to CSS is easy. Consider the following example of Sass code.
/*define a variable for the primary color*/
$primary : #434433;
/*use the lighten() function to determine the disabled value, and define it as a variable*/
$disabled: lighten($primary, 20%);
.menu-item {
color: $primary;
.disabled-menu-item {
color: $disabled;
}
Now, Saas transpiler will generate the following CSS for the above Sass code.
.menu-item {
color: #434433;
.disabled-menu-item {
color: #76785A;
}
As you can see, variables are replaced by values in the CSS. Thus, Sass makes it easy to create and maintain CSS files for your application.
Learn to install Sass in the next chapter.