Sass Script
So far we've looked at Sass as a way of writing CSS that's more concise and easier to read. But the Sass transpiler can do a whole lot more. It's not quite a full-fledged programming language, it's missing some of the constructs we expect from modern languages. But, it does provide some basic scripting functionality, including arithmetic operators, flow of control directives, and some very handy functions.
SassScript is a script which allows us to compute CSS selector, property or value using Sass expression. We'll look at expressions in this chapter and explore data types, SassScript control directives, operations and functions in the next few chapters. All of these can be use in SassScript.
Sass Expression
When you're using SassScript, you're still writing CSS. You have access to the CSS extensions we explored in the Syntax chapter, but you'll still be using the basic structure of a CSS rule:
<selector> { <property>: <<span className="kwrd">value</span>>; <property>: <<span className="kwrd">value</span>>; ... }
That doesn't change. What does change is that you can replace <selector>, <property> or <value> with an expression. A Sass expression is some combination of values and operators that the Sass transpiler will evaluate before outputting the final CSS.
The variables we've already explored are an important example of a SassScript expression. When the transpiler sees the following code:
$dark-green: #0f5a0c;
p {
color: $dark-green;
}
It evaluates the variable $dark-green
and replaces the result, #0f5a0c in this case, in the CSS it outputs:
p {
color: $0f5a0c;
}
But SassScript expressions can be little more complex than a variable. They can include operations as shown below. (we'll explore in detail in the operators chapter.)
$text-size: 25px;
p {
font-size: $text-size * 1.20;
}
This example will evaluate both the variable $text-size
and the operator * (multiply) and output the following CSS:
p {
font-size = 30px;
}
You can also use one of the functions that SassScript provides (we'll explore these in the Functions chapter):
p {
font-color = rgb(135, 204, 166);
}
The rgb()
SassScript function generates a hex color value from the provided red green and blue colors, so the transpiler will output:
p {
font-color = #87cca6;
}
In each case, the transpiler is doing essentially the same thing: It evaluates the expression, which may include variables, operators and functions in any combination, and outputs simple CSS values.
Using the SassScript Interpreter
When you're working with SassScript, it's handy to be able to execute expressions without going through the transpiling process. Sass provides a command-line interpreter, available in the command window.
NOTE: On Windows, make sure to use the command window installed when you installed Ruby.
Load the Sass interpreter with the command:
sass -i
Your command prompt will change from the default (which varies, depending on which operating system you're using) to a >>. The following is a sass interpreter in windows platform.
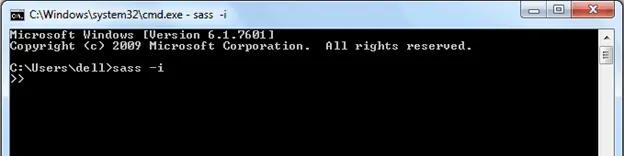
Now you can type any expression, and the result will be displayed in the window. You can even use variables, but the interpreter will only remember them for a single session. If you close the command window, the interpreter won't remember the variable values next time.
Why don't you give it a try by entering the example expressions from this chapter? Remember, you want to just enter the expression, not the CSS, and don't add a semicolon at the end. For example, enter rgb(135, 204, 166)
not font-color = rgb(135, 204, 166);
, which won't work.
Learn about the data types in the next chapter.