Here you will learn how to parse JSON string to class object in C#.
JSON is known as Javascript Object Notation used for storing and transferring data. In the C# application, you often need to convert JSON string data to class objects.
For example, assume that you have the following JSON string:
"{\"DeptId\": 101, \"DepartmentName\": \"IT\"}";
Now, to convert the above string to a class object, the name of the data properties in the string must match with the name of the class properties. To convert the above JSON string, the class should be as below:
public class Department{
public int DeptId { get; set; }
public string DepartmentName { get; set; }
}
Notice that the above properties of the above class match with the properties of a JSON string. Therefore, any change in the name would result in an exception.
The .NET Core 3.0 and later versions include the built-in class JsonSerializer
in the System.Text.Json
namespace that provides functionality for serializing and deserializing from JSON.
The .NET 4.x framework does not provide any built-in JsonSerializer
class that converts objects to JSON.
You have to install the NuGet package Microsoft.Extensions.Configuration.Json
in your project to include the System.Text.Json.JsonSerializer
to your project which can be used to convert objects to JSON and vice-versa.
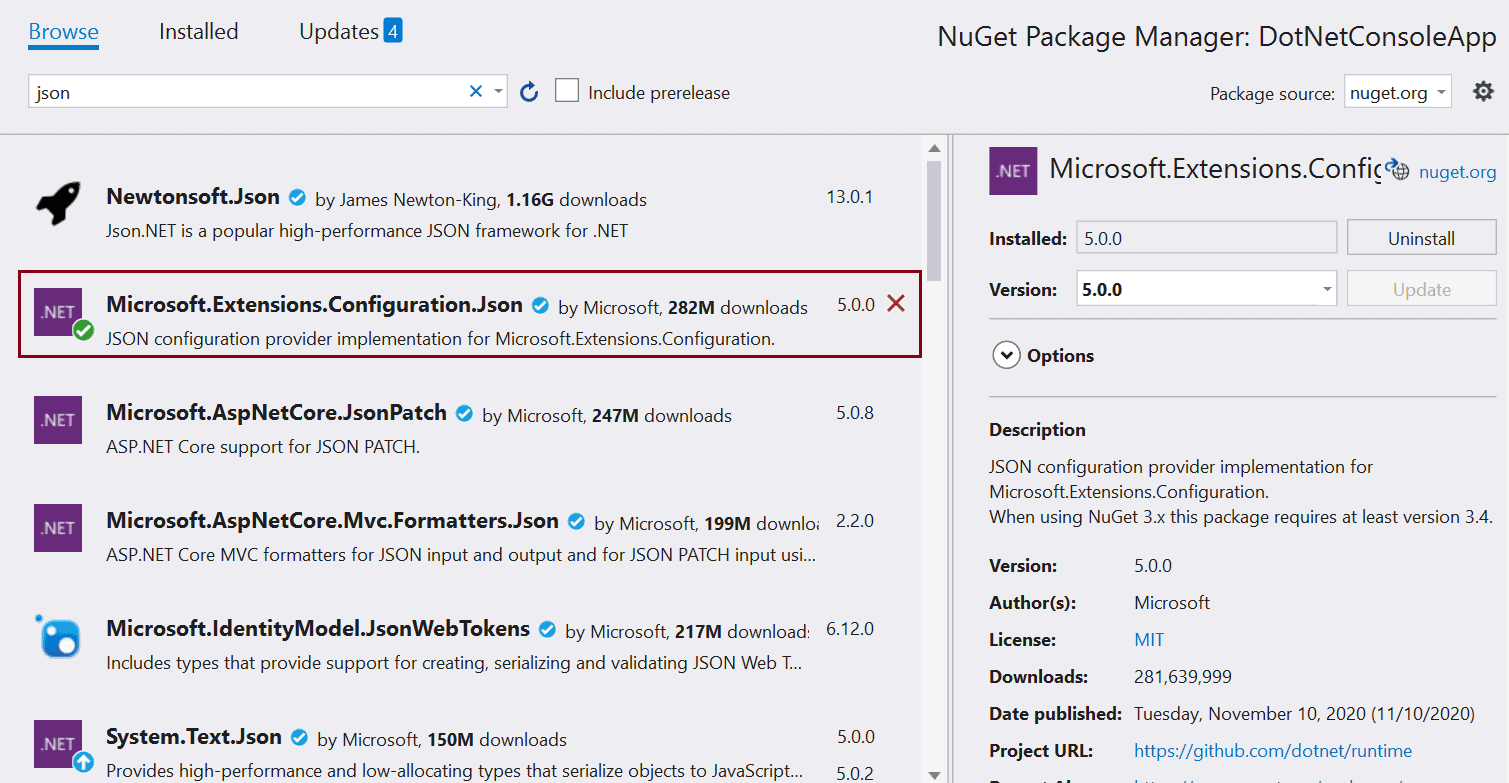
Deserialization is the process of parsing a string into an object of a specific type. The JsonSerializer.Deserialize()
method converts a JSON string into an object of the type specified by a generic type parameter.
Syntax:
public static TValue? Deserialize<TValue> (string json,
JsonSerializerOptions? options = default);
The following example shows how to parse a JSON string using the JsonSerializer.Deserialize
()
method:
string jsonData = "{\"DeptId\": 101, \"DepartmentName\": \"IT\"}";
Department deptObj = JsonSerializer.Deserialize<Department>(jsonData);
Console.WriteLine("Department Id: {0}", deptObj.DeptId);
Console.WriteLine("Department Name: {0}", deptObj.DepartmentName);
Department Name is: IT
Convert JSON Array String to List
Many times the JSON string contains an array to store multiple data. This can be converted to an array or list of objects in C#. The following example shows how to parse JSON array to C# list collection.
string jsonArray = "[{\"DeptId\": 101,\"DepartmentName\":\"IT\" }, {\"DeptId\": 102,\"DepartmentName\":\"Accounts\" }]";
var deptList = JsonSerializer.Deserialize<IList<Department>>(jsonArray);
foreach(var dept in deptList)
{
Console.WriteLine("Department Id is: {0}", dept.DeptId);
Console.WriteLine("Department Name is: {0}", dept.DepartmentName);
}
Department Name is: IT
Department Id is: 102
Department Name is: Accounts
Convert JSON String to Object in AJAX Application
Use the JavaScriptSerializer
class to provide serialization and deserialization functionality for AJAX-enabled ASP.NET web applications.
The JavaScriptSerializer.Deserialize()
method converts the specified JSON string to the type of the specified generic parameter object.
The following example shows how to parse JSON string using JavaScriptSerializer.Deserialize()
method.
using System;
using System.Collections.Generic;
using System.Web.UI;
using System.Web.Script.Serialization;
namespace MyWebApplication
{
public partial class _Default : Page
{
protected void Page_Load(object sender, EventArgs e)
{
string jsonDept = @"{'DeptId': '101', 'DepartmentName': 'IT'}";
var serializer = new JavaScriptSerializer();
Department deptObj = new serializer.Deserialize<Department>(jsonDept);
}
}
public class Department
{
public int DeptId { get; set; }
public string DepartmentName { get; set; }
}
}
Learn more about System.Web.Script.Serialization.JavaScriptSerializer.
Thus, convert the JSON string to class object in C# using the JsonSerializer.Deserialize()
method.
- How to get the sizeof a datatype in C#?
- Difference between String and StringBuilder in C#
- Static vs Singleton in C#
- Difference between == and Equals() Method in C#
- Asynchronous programming with async, await, Task in C#
- How to loop through an enum in C#?
- Generate Random Numbers in C#
- Difference between Two Dates in C#
- Convert int to enum in C#
- BigInteger Data Type in C#
- Convert String to Enum in C#
- Convert an Object to JSON in C#
- DateTime Formats in C#
- How to convert date object to string in C#?
- Compare strings in C#
- How to count elements in C# array?
- Difference between String and string in C#.
- How to get a comma separated string from an array in C#?
- Boxing and Unboxing in C#
- How to convert string to int in C#?