Python Syntax
Here, you will learn the basic syntax of Python 3.
Display Output
The print() funtion in Python displays an output to a console or to the text stream file.
You can pass any type of data to the print()
function to be displayed on the console.
print('Hello World!')
print(1234)
print(True)
In Python shell, it echoes the value of any Python expression.

The print()
function can also display the values of one or more variables separated by a comma.
name="Ram"
print(name) # display single variable
age=21
print(name, age)# display multiple variables
print("Name:", name, ", Age:",age) # display formatted output
By default, a single space ' '
acts as a separator between values. However, any other character can be used by providing a sep
parameter.
Visit the print() funtion for more information.
Getting User's Input
The input() function is used to get the user's input. It reads the key strokes as a string object which can be referred to by a variable having a suitable name.

Note that the blinking cursor waits for the user's input. The user enters his input and then hits Enter. This will be captured as a string.
In the above example, the input()
function takes the user's input from the next line, e.g. 'Steve' in this case.
input()
will capture it and assign it to a name
variable.
The name
variable will display whatever the user has provided as the input.
The input()
function has an optional string parameter that acts as a prompt for the user.
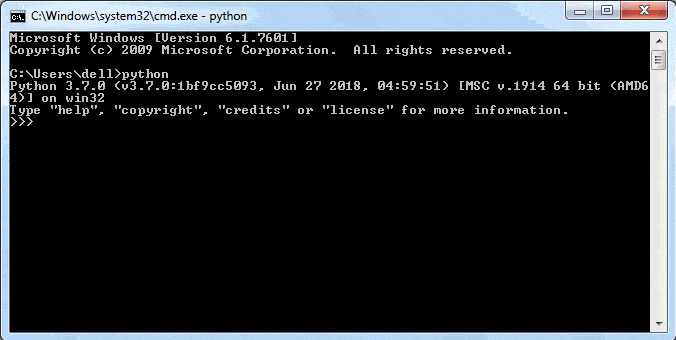
The input()
function always reads the input as a string, even if comprises of digits.
Visit input() function for more information.
Python Statements
Python statement ends with the token NEWLINE character (carriage return). It means each line in a Python script is a statement. The following Python script contains three statements in three separate lines.
print('id: ', 1)
print('First Name: ', 'Steve')
print('Last Name: ', 'Jobs')
Use backslash character \
to join a statement span over multiple lines, as shown below.
print('id: ',\
1)
print('First Name: ',\
'Ste\
ve')
print('Last \
Name: ',
'Jobs')
Expressions in parentheses ()
, square brackets [ ]
, or curly braces { }
can be spread over multiple lines without using backslashes.
list = [1, 2, 3, 4
5, 6, 7, 8,
9, 10, 11, 12]
Please note that the backslash character spans a single line in one logical line and multiple physical lines, but not the two different statements in one logical line.
Use the semicolon ;
to separate multiple statements in a single line.
print('id: ', 1);print('First Name: ', 'Steve');print('Last Name: ', 'Jobs')
Code Comments in Python
In a Python script, the symbol # indicates the start of a comment line. It is effective till the end of the line in the editor.
# this is a comment
print("Hello World")
print("Welcome to Python Tutorial") #comment after a statement.
In Python, there is no provision to write multi-line comments, or a block comment. For multi-line comments, each line should have the #
symbol at the start.
A triple quoted multi-line string is also treated as a comment if it is not a docstring of the function or the class.
'''
comment1
comment2
comment3
'''
Visit PEP 8 style Guide for Python Code for more information.
Indentation in Python
Leading space or tab at the beginning of the line is considered as indentation level of the line, which is used to determine the group of statements. Statements with the same level of indentation considered as a group or block.
For example, functions, classes, or loops in Python contains a block of statements to be executed.
Other programming languages such as C# or Java use curly braces { }
to denote a block of code.
Python uses indentation (a space or a tab) to denote a block of statements.
Indentation Rules
- Use the colon : to start a block and press Enter.
- All the lines in a block must use the same indentation, either space or a tab.
- Python recommends four spaces as indentation to make the code more readable. Do not mix space and tab in the same block.
- A block can have inner blocks with next level indentation.
The following example demonstrates if elif blocks:
if 10 > 5: # 1st block starts
print("10 is greater than 5") # 1st block
if 20 > 10: # 1st block
print("20 is greater than 10") # inner block
else: # 2nd block starts
print("10 is less than 5") # 2nd block
A function contains all the same level indented statements. The following function contains a block with two statements.
def SayHello(name):
print("Hello ", name)
print("This is the second statement of the SayHello() function")
print("This is the last statement of the SayHello() function")
print("This is not the part of the SayHello() function")
#calling a function
SayHello("Abdul")
The following example illustrates the use of indents in Python shell:

As you can see, in the Python shell, the SayHello()
function block started after :
and pressing Enter.
It then displayed ... to mark the block. Use four space (even a single space is ok) or a tab for indent and then write a statement.
To end the block, press Enter two times.
Python Naming Convetions
The Python program can contain variables, functions, classes, modules, packages, etc. Identifier is the name given to these programming elements. An identifier should start with either an alphabet letter (lower or upper case) or an underscore (_). After that, more than one alphabet letter (a-z or A-Z), digits (0-9), or underscores may be used to form an identifier. No other characters are allowed.
- Identifiers in Python are case sensitive, which means variables named
age
andAge
are different. -
Class names should use the TitleCase convention. It should begin with an uppercase alphabet letter e.g.
MyClass
,Employee
,Person
. -
Function names should be in lowercase. Multiple words should be separated by underscores, e.g.
add(num)
,calculate_tax(amount)
. - Variable names in the function should be in lowercase e.g.,
x
,num
,salary
. - Module and package names should be in lowercase e.g.,
mymodule
,tax_calculation
. Use underscores to improve readability. - Constant variable names should be in uppercase e.g.,
RATE
,TAX_RATE
. - Use of one or two underscore characters when naming the instance attributes of a class.
- Two leading and trailing underscores are used in Python itself for a special purpose, e.g. __add__, __init__, etc.
Visit PEP 8 - Prescriptive Naming Conventions for more information.