C# for Loop
Here, you will learn how to execute a statement or code block multiple times using the for loop, structure of the for loop, nested for loops, and how to exit from the for loop.
The for
keyword indicates a loop in C#. The for
loop executes a block of statements repeatedly until the specified condition returns false.
for (initializer; condition; iterator) { //code block }
The for
loop contains the following three optional sections, separated by a semicolon:
Initializer: The initializer section is used to initialize a variable that will be local to a for loop and cannot be accessed outside loop. It can also be zero or more assignment statements, method call, increment, or decrement expression e.g., ++i or i++, and await expression.
Condition: The condition is a boolean expression that will return either true or false. If an expression evaluates to true, then it will execute the loop again; otherwise, the loop is exited.
Iterator: The iterator defines the incremental or decremental of the loop variable.
The following for loop executes a code block 10 times.
for(int i = 0; i < 10; i++)
{
Console.WriteLine("Value of i: {0}", i);
}
Value of i: 1
Value of i: 2
Value of i: 3
Value of i: 4
Value of i: 5
Value of i: 6
Value of i: 7
Value of i: 8
Value of i: 9
In the above example, int i = 0
is an initializer where we define an int variable i
and initialize it with 0.
The second section is the condition expression i < 10
, if this condition returns true
then it will execute a code block. After executing the code block, it will go to the third section, iterator.
The i++
is an incremental statement that increases the value of a loop variable i
by 1. Now, it will check the conditional expression again and repeat the same thing until conditional expression returns false
.
The below figure illustrates the execution steps of the for
loop.
The below figure illustrates the execution steps of the for
loop.
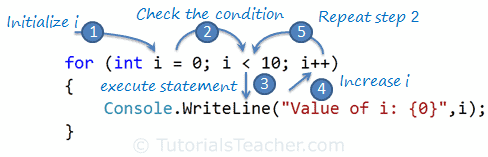
If a code block only contains a single statement, then you don't need to wrap it inside curly brackets { }
, as shown below.
for(int i = 0; i < 10; i++)
Console.WriteLine("Value of i: {0}", i);
An Initializer, condition, and iterator sections are optional. You can initialize a variable before for
loop, and condition and iterator can be defined inside a code block, as shown below.
int i = 0;
for(;;)
{
if (i < 10)
{
Console.WriteLine("Value of i: {0}", i);
i++;
}
else
break;
}
Value of i: 1
Value of i: 2
Value of i: 3
Value of i: 4
Value of i: 5
Value of i: 6
Value of i: 7
Value of i: 8
Value of i: 9
Since all three sections are optional in the for
loop, be careful in defining a condition and iterator. Otherwise, it will be an infinite loop that will never end the loop.
for ( ; ; )
{
Console.Write(1);
}
The control variable for the for loop can be of any numeric data type, such as double, decimal, etc.
for (double d = 1.01D; d < 1.10; d+= 0.01D)
{
Console.WriteLine("Value of i: {0}", d);
}
Value of i: 1.02
Value of i: 1.03
Value of i: 1.04
Value of i: 1.05
Value of i: 1.06
Value of i: 1.07
Value of i: 1.08
Value of i: 1.09
The steps part in a for loop can either increase or decrease the value of a variable.
for(int i = 10; i > 0; i--)
{
Console.WriteLine("Value of i: {0}", i);
}
Value of i: 9
Value of i: 8
Value of i: 7
Value of i: 6
Value of i: 5
Value of i: 4
Value of i: 3
Value of i: 2
Value of i: 1
Exit the for Loop
You can also exit from a for loop by using the break
keyword.
for (int i = 0; i < 10; i++)
{
if( i == 5 )
break;
Console.WriteLine("Value of i: {0}", i);
}
Value of i: 1
Value of i: 2
Value of i: 3
Value of i: 4
Multiple Expressions
A for
loop can also include multiple initializer and iterator statements separated by comma, as shown below.
for (int i = 0, j = 0; i+j < 5; i++, j++)
{
Console.WriteLine("Value of i: {0}, J: {1} ", i,j);
}
Value of i: 1, J: 1
Value of i: 2, J: 2
A for
loop can also contain statements as an initializer and iterator.
int i = 0, j = 5;
for (Console.WriteLine($"Initializer: i={i}, j={j}");
i++ < j--;
Console.WriteLine($"Iterator: i={i}, j={j}"))
{
}
Iterator: i=1, j=4
Iterator: i=2, j=3
Iterator: i=3, j=2
Nested for Loop
C# allows a for loop inside another for loop.
for (int i = 0; i < 2; i++)
{
for(int j =i; j < 4; j++)
Console.WriteLine("Value of i: {0}, J: {1} ", i,j);
}
Value of i: 0, J: 1
Value of i: 0, J: 2
Value of i: 0, J: 3
Value of i: 1, J: 1
Value of i: 1, J: 2
Value of i: 1, J: 3